Руководство C# String и StringBuilder
1. Иерархия наследования
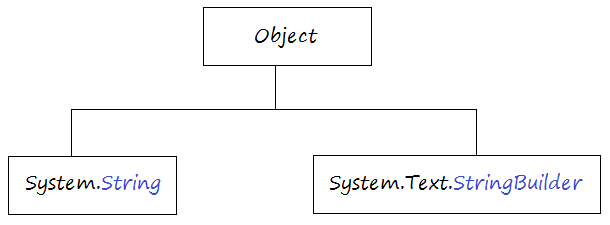
При работе с текстовыми данными CSharp предоставляет вам 2 класса String и StringBuilder. При работе с большими данными вы должны использовать StringBuilder для эффективной оптимизации. В принципе, эти 2 класса имеют много общего.
- String является неизменяемым (immutable), более подробная информация об этой концепции содержится в статье, и не допускает существования подкласса.
- StringBuilder изменчив (mutable).
2. Понятия mutable & immutable
Рассмотрим иллюстрированный пример:
MutableClassExample.cs
namespace StringTutorial
{
// Это класс с 1 полем: 'Value'.
// Если у вас есть объект данного класса
// Вы можете прикрепить новое значение для поля 'Value'
// через метод SetNewValue(int).
// Таким образом это изменный класс (mutable).
class MutableClassExample
{
private int Value;
public MutableClassExample(int value)
{
this.Value = value;
}
public void SetNewValue(int newValue)
{
this.Value = newValue;
}
}
}
ImmutableClassExample.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace StringTutorial
{
// Это класс имеющий 2 поля (field): 'Value' & 'Name'.
// Если вы хотите получить объект данного класса
// Вы не можете прикрепить новое значение для 'Value','Name' снаружи,
// Это значит данный класс является неизменным (immutable)
class ImmutableClassExample
{
private int Value;
private String Name;
public ImmutableClassExample(String name, int value)
{
this.Value = value;
this.Name = name;
}
public String GetName()
{
return Name;
}
public int GetValue()
{
return Value;
}
}
}
String - неизменяемый класс. String включает различные поля, такие как длина (Length), но эти значения не могут быть изменены.
3. String и string
В C# иногда вы видите, что String и string используются параллельно. На самом деле, они не имеют никакой разницы, string может рассматриваться как псевдоним (alias) для System.String (полное имя, включая пространство имен класса String).
В таблице ниже приведен полный список псевдонимов общих классов.
В таблице ниже приведен полный список псевдонимов общих классов.
Alias | Class |
object | System.Object |
string | System.String |
bool | System.Boolean |
byte | System.Byte |
sbyte | System.SByte |
short | System.Int16 |
ushort | System.UInt16 |
int | System.Int32 |
uint | System.UInt32 |
long | System.Int64 |
ulong | System.UInt64 |
float | System.Single |
double | System.Double |
decimal | System.Decimal |
char | System.Char |
4. String
String это очень важный в CSharp, и любой, кто начинает с CSharp, использует команду Console.WriteLine(), чтобы распечатать String на экран Console. Многие не имеют понятия, что String является неизменяемым (immutable) и опечатанным (не позволяет иметь подклассы). Каждая модификация в String создает новый String объект.
** String **
[SerializableAttribute]
[ComVisibleAttribute(true)]
public sealed class String : IComparable, ICloneable, IConvertible,
IEnumerable, IComparable<string>, IEnumerable<char>, IEquatable<string>
Методы String
Вы можете посмотреть методы String по ссылке:
Ниже приведён список некоторых распространённых методов String:
Some String methods
public bool EndsWith(string value)
public bool EndsWith(string value, StringComparison comparisonType)
public bool Equals(string value)
public int IndexOf(char value)
public int IndexOf(char value, int startIndex)
public int IndexOf(string value, int startIndex, int count)
public int IndexOf(string value, int startIndex, StringComparison comparisonType)
public int IndexOf(string value, StringComparison comparisonType)
public string Insert(int startIndex, string value)
public int LastIndexOf(char value)
public int LastIndexOf(char value, int startIndex)
public int LastIndexOf(char value, int startIndex, int count)
public int LastIndexOf(string value)
public int LastIndexOf(string value, int startIndex)
public int LastIndexOf(string value, int startIndex, int count)
public int LastIndexOf(string value, int startIndex, int count, StringComparison comparisonType)
public int LastIndexOf(string value, int startIndex, StringComparison comparisonType)
public int LastIndexOf(string value, StringComparison comparisonType)
public int LastIndexOfAny(char[] anyOf)
public int LastIndexOfAny(char[] anyOf, int startIndex)
public int LastIndexOfAny(char[] anyOf, int startIndex, int count)
public int IndexOf(string value, int startIndex, int count, StringComparison comparisonType)
public string Replace(char oldChar, char newChar)
public string Replace(string oldValue, string newValue)
public string[] Split(params char[] separator)
public string[] Split(char[] separator, int count)
public string[] Split(char[] separator, int count, StringSplitOptions options)
public string[] Split(char[] separator, StringSplitOptions options)
public string[] Split(string[] separator, StringSplitOptions options)
public bool StartsWith(string value)
public bool StartsWith(string value, bool ignoreCase, CultureInfo culture)
public bool StartsWith(string value, StringComparison comparisonType)
public string Substring(int startIndex)
public string Substring(int startIndex, int length)
public char[] ToCharArray()
public char[] ToCharArray(int startIndex, int length)
public string ToLower()
public string ToLower(CultureInfo culture)
public string ToLowerInvariant()
public override string ToString()
public string ToUpper()
public string ToUpper(CultureInfo culture)
public string ToUpperInvariant()
public string Trim()
public string Trim(params char[] trimChars)
public string TrimEnd(params char[] trimChars)
public string TrimStart(params char[] trimChars)
5. StringBuilder
В C# каждая модификация в результате String создает новый объект String. Между тем StringBuilder содержит в себе массив символов, этот массив будет автоматически заменен на больший массив, если это необходимо, и скопирует символы из старого массива. Если вам необходимо объединить несколько строк много раз, вы должны использовать StringBuilder, это помогает повысить эффективность программы. Но это не обязательно, если вы только объединяете (concatenate) несколько строк, вы не должны злоупотреблять StringBuilder в этом случае.
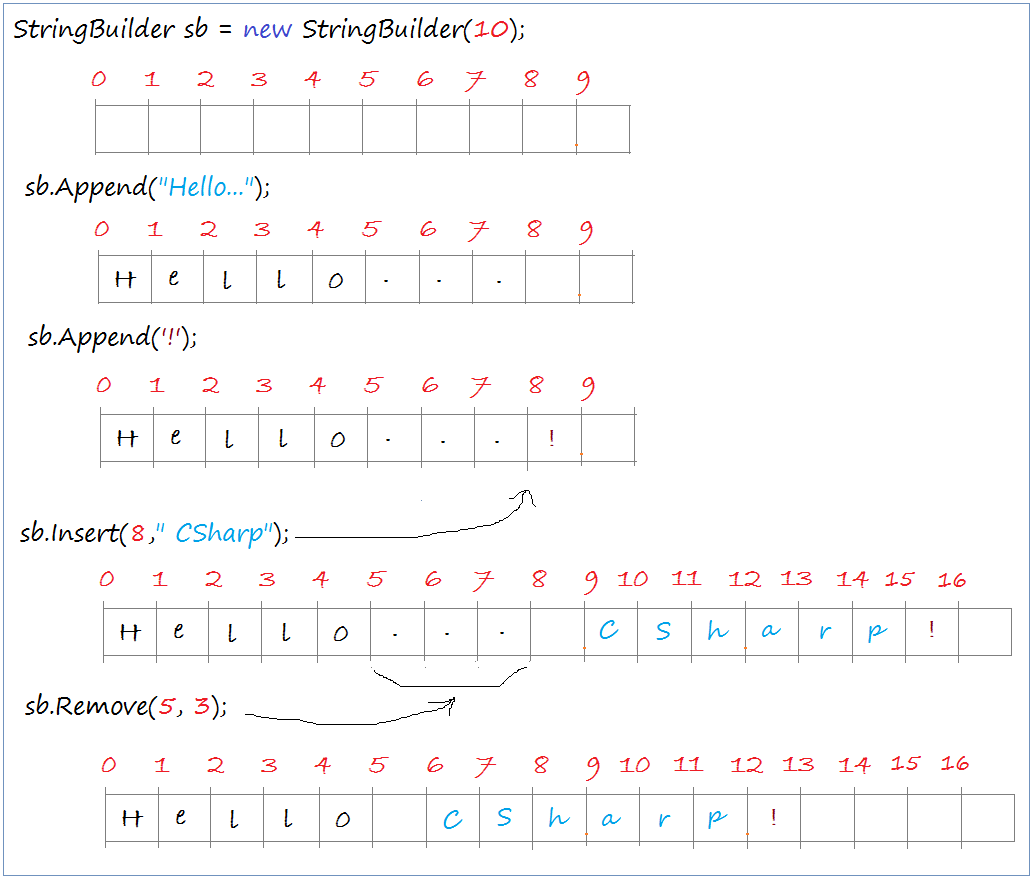
StringBuilderDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace StringTutorial
{
class StringBuilderDemo
{
public static void Main(string[] args)
{
// Создать объект StringBuilder
// с объемом (capacity) содержащим 10 символов.
StringBuilder sb = new StringBuilder(10);
// Соединить подстроку.
sb.Append("Hello...");
Console.WriteLine("- sb after appends a string: " + sb);
// Соединить символ.
char c = '!';
sb.Append(c);
Console.WriteLine("- sb after appending a char: " + sb);
// Вставить String в индекс 5.
sb.Insert(8, " CSharp");
Console.WriteLine("- sb after insert string: " + sb);
// Удалить подстроку начиная с индекса 5, с 3 символами.
sb.Remove(5, 3);
Console.WriteLine("- sb after remove: " + sb);
// Получить string в StringBuilder.
String s = sb.ToString();
Console.WriteLine("- String of sb: " + s);
Console.Read();
}
}
}
Результаты выполнения примера:
- sb after appends a string: Hello...
- sb after appending a char: Hello...!
- sb after insert string: Hello... CSharp!
- sb after remove: Hello CSharp!
- String of sb: Hello CSharp!
Pуководства C#
- Наследование и полиморфизм в C#
- Что мне нужно для начала работы с C#?
- Быстрый обучение C# для начинающих
- Установите Visual Studio 2013 в Windows
- Абстрактный класс и Interface в C#
- Установите Visual Studio 2015 в Windows
- Сжатие и декомпрессия в C#
- Руководство по программированию многопоточности C#
- Руководство C# Delegate и Event
- Установите AnkhSVN в Windows
- Программирование C# для группы использующей Visual Studio и SVN
- Установить .Net Framework
- Access Modifier (Модификатор доступа) в C#
- Руководство C# String и StringBuilder
- Руководство C# Property
- Руководство C# Enum
- Руководство C# Structure
- Руководство C# Generics
- Обработка исключений для C#
- Руководство C# Date Time
- Манипулирование файлами и каталогами в C#
- Руководство CSharp Streams - двоичные потоки в C#
- Руководство Регулярное выражение C#
- Подключиться к базе данных SQL Server в C#
- Работа с базой данных SQL Server на C#
- Подключиться к базе данных MySQL в C#
- Работа с базой данных MySQL на C#
- Подключиться к базе данных Oracle в C# без Oracle Client
- Работа с базой данных Oracle на C#
Show More