Руководство C# Date Time
1. Классы связанные с Date, Time в C#
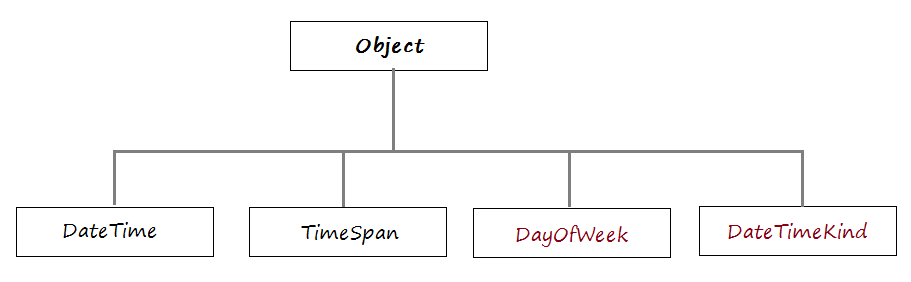
public DateTime(int year, int month, int day)
public DateTime(int year, int month, int day, Calendar calendar)
public DateTime(int year, int month, int day, int hour, int minute, int second)
public DateTime(int year, int month, int day, int hour, int minute, int second, Calendar calendar)
public DateTime(int year, int month, int day, int hour, int minute, int second, DateTimeKind kind)
public DateTime(int year, int month, int day, int hour, int minute, int second, int millisecond)
public DateTime(int year, int month, int day, int hour,
int minute, int second, int millisecond, Calendar calendar)
public DateTime(int year, int month, int day, int hour,
int minute, int second, int millisecond, Calendar calendar, DateTimeKind kind)
public DateTime(int year, int month, int day, int hour,
int minute, int second, int millisecond, DateTimeKind kind)
public DateTime(long ticks)
public DateTime(long ticks, DateTimeKind kind)
// Объект описывает настоящее время.
DateTime now = DateTime.Now;
Console.WriteLine("Now is "+ now);
2. Атрибуты DateTime
Property | Data Type | Description |
Date | DateTime | Gets the date component of this instance. |
Day | int | Gets the day of the month represented by this instance. |
DayOfWeek | DayOfWeek | Gets the day of the week represented by this instance. |
DayOfYear | int | Gets the day of the year represented by this instance. |
Hour | int | Gets the hour component of the date represented by this instance. |
Kind | DateTimeKind | Gets a value that indicates whether the time represented by this instance is based on local time, Coordinated Universal Time (UTC), or neither. |
Millisecond | int | Gets the milliseconds component of the date represented by this instance. |
Minute | int | Gets the minute component of the date represented by this instance. |
Month | int | Gets the month component of the date represented by this instance. |
Now | DateTime | Gets a DateTime object that is set to the current date and time on this computer, expressed as the local time. |
Second | int | Gets the seconds component of the date represented by this instance. |
Ticks | long | Gets the number of ticks that represent the date and time of this instance. |
TimeOfDay | TimeSpan | Gets the time of day for this instance. |
Today | DateTime | Gets the current date. |
UtcNow | DateTime | Gets a DateTime object that is set to the current date and time on this computer, expressed as the Coordinated Universal Time (UTC). |
Year | int | Gets the year component of the date represented by this instance. |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class DateTimePropertiesExample
{
public static void Main(string[] args)
{
// Создать объект DateTime (год, месяц, день, час, минута, секунда).
DateTime aDateTime = new DateTime(2005, 11, 20, 12, 1, 10);
// Распечатать информацию:
Console.WriteLine("Day:{0}", aDateTime.Day);
Console.WriteLine("Month:{0}", aDateTime.Month);
Console.WriteLine("Year:{0}", aDateTime.Year);
Console.WriteLine("Hour:{0}", aDateTime.Hour);
Console.WriteLine("Minute:{0}", aDateTime.Minute);
Console.WriteLine("Second:{0}", aDateTime.Second);
Console.WriteLine("Millisecond:{0}", aDateTime.Millisecond);
// Enum {Monday, Tuesday,... Sunday}
DayOfWeek dayOfWeek = aDateTime.DayOfWeek;
Console.WriteLine("Day of Week:{0}", dayOfWeek );
Console.WriteLine("Day of Year: {0}", aDateTime.DayOfYear);
// Объект только описывает время (час минута секунда,..)
TimeSpan timeOfDay = aDateTime.TimeOfDay;
Console.WriteLine("Time of Day:{0}", timeOfDay);
// Конвертирует вTicks (1 секунда = 10.000.000 Ticks)
Console.WriteLine("Tick:{0}", aDateTime.Ticks);
// {Local, Itc, Unspecified}
DateTimeKind kind = aDateTime.Kind;
Console.WriteLine("Kind:{0}", kind);
Console.Read();
}
}
}
Day:20
Month:11
Year:2005
Hour:12
Minute:1
Second:10
Millisecond:0
Day of Week:Sunday
Day of Year: 325
Time of Day:12:01:10
Tick:632680848700000000
3. Добавить и убавить время
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class AddSubtractExample
{
public static void Main(string[] args)
{
// Настоящее время.
DateTime aDateTime = DateTime.Now;
Console.WriteLine("Now is " + aDateTime);
// Период времени.
// 1 час + 1 минута
TimeSpan aInterval = new System.TimeSpan(0, 1, 1, 0);
// Добавить период времени.
DateTime newTime = aDateTime.Add(aInterval);
Console.WriteLine("After add 1 hour, 1 minute: " + newTime);
// Убавить период времени.
newTime = aDateTime.Subtract(aInterval);
Console.WriteLine("After subtract 1 hour, 1 minute: " + newTime);
Console.Read();
}
}
}
Now is 12/8/2015 10:52:03 PM
After add 1 hour, 1 minute: 12/8/2015 11:53:03 PM
After subtract 1 hour, 1 minute: 12/8/2015 9:51:03 PM
- AddYears
- AddDays
- AddMinutes
- ...
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class AddSubtractExample2
{
public static void Main(string[] args)
{
// Настоящее время.
DateTime aDateTime = DateTime.Now;
Console.WriteLine("Now is " + aDateTime);
// Добавить 1 год
DateTime newTime = aDateTime.AddYears(1);
Console.WriteLine("After add 1 year: " + newTime);
// Убавить 1 час
newTime = aDateTime.AddHours(-1);
Console.WriteLine("After add -1 hour: " + newTime);
Console.Read();
}
}
}
Now is 12/8/2015 11:28:34 PM
After add 1 year: 12/8/2016 11:28:34 PM
After add -1 hour: 12/8/2015 10:28:34 PM
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class FirstLastDayDemo
{
public static void Main(string[] args)
{
Console.WriteLine("Today is " + DateTime.Today);
DateTime yesterday = GetYesterday();
Console.WriteLine("Yesterday is " + yesterday);
// Первый день февраля 2015 года
DateTime aDateTime = GetFistDayInMonth(2015, 2);
Console.WriteLine("First day of 2-2015: " + aDateTime);
// Последний день февраля 2015 года
aDateTime = GetLastDayInMonth(2015, 2);
Console.WriteLine("Last day of 2-2015: " + aDateTime);
// Первый день 2015 года
aDateTime = GetFirstDayInYear(2015);
Console.WriteLine("First day year 2015: " + aDateTime);
// Последний день 2015 года
aDateTime = GetLastDayInYear(2015);
Console.WriteLine("Last day year 2015: " + aDateTime);
Console.Read();
}
// Возвращает вчерашний день.
public static DateTime GetYesterday()
{
// Сегодняшний день.
DateTime today = DateTime.Today;
// Убавляет 1 день.
return today.AddDays(-1);
}
// Возвращает первый день в году
public static DateTime GetFirstDayInYear(int year)
{
DateTime aDateTime = new DateTime(year, 1, 1);
return aDateTime;
}
// Возвращает последний день в году
public static DateTime GetLastDayInYear(int year)
{
DateTime aDateTime = new DateTime(year +1, 1, 1);
// Убавляет 1 день.
DateTime retDateTime = aDateTime.AddDays(-1);
return retDateTime;
}
// Возвращает первый день в месяце
public static DateTime GetFistDayInMonth(int year, int month)
{
DateTime aDateTime = new DateTime(year, month, 1);
return aDateTime;
}
// Возвращает последний день в месяце
public static DateTime GetLastDayInMonth(int year, int month)
{
DateTime aDateTime = new DateTime(year, month, 1);
// Прибавляет 1 месяц и убавляет 1 день.
DateTime retDateTime = aDateTime.AddMonths(1).AddDays(-1);
return retDateTime;
}
}
}
Today is 12/9/2015 12:00:00 AM
Yesterday is 12/8/2015 12:00:00 AM
First day of 2-2015: 2/1/2015 12:00:00 AM
Last day of 2-2015: 2/28/2015 12:00:00 AM
First day year 2015: 2/1/2015 12:00:00 AM
Last day year 2015: 12/31/2015 12:00:00 AM
4. Интервал времени
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class IntervalDemo
{
public static void Main(string[] args)
{
// Настоящее время.
DateTime aDateTime = DateTime.Now;
// Во время 2000 года
DateTime y2K = new DateTime(2000,1,1);
// Период времени от 2000 до данного момента.
TimeSpan interval = aDateTime.Subtract(y2K);
Console.WriteLine("Interval from Y2K to Now: " + interval);
Console.WriteLine("Days: " + interval.Days);
Console.WriteLine("Hours: " + interval.Hours);
Console.WriteLine("Minutes: " + interval.Minutes);
Console.WriteLine("Seconds: " + interval.Seconds);
Console.Read();
}
}
}
Interval from Y2K to Now: 5820.23:51:08.1194036
Days: 5820
Hours: 23
Minutes: 51
Seconds: 8
5. Сравнить 2 объекта DateTime
// Если значение < 0 значит firstDateTime раньше (стоит впереди)
// Если значение > 0 значит secondDateTime раньше (стоит впереди).
// Если значение = 0 значит 2 этих объекта одинаковы по времени.
public static int Compare(DateTime firstDateTime, DateTime secondDateTime);

using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class CompareDateTimeExample
{
public static void Main(string[] args)
{
// Настоящее время.
DateTime firstDateTime = new DateTime(2000, 9, 2);
DateTime secondDateTime = new DateTime(2011, 1, 20);
int compare = DateTime.Compare(firstDateTime, secondDateTime);
Console.WriteLine("First DateTime: " + firstDateTime);
Console.WriteLine("Second DateTime: " + secondDateTime);
Console.WriteLine("Compare value: " + compare);// -1
if (compare < 0)
{
// firstDateTime раньше secondDateTime.
Console.WriteLine("firstDateTime is earlier than secondDateTime");
}
else
{
// firstDateTime позже secondDateTime
Console.WriteLine("firstDateTime is laster than secondDateTime");
}
Console.Read();
}
}
}
First DateTime: 9/2/2000 12:00:00 AM
Second DateTime: 1/20/2011 12:00:00 AM
Compare value: -1
firstDateTime is earlier than secondDateTime
6. Стандартный формат DateTime
- Конвертирует значение этого экземпляра (datetime) в массив строк, форматированные по стандарту.
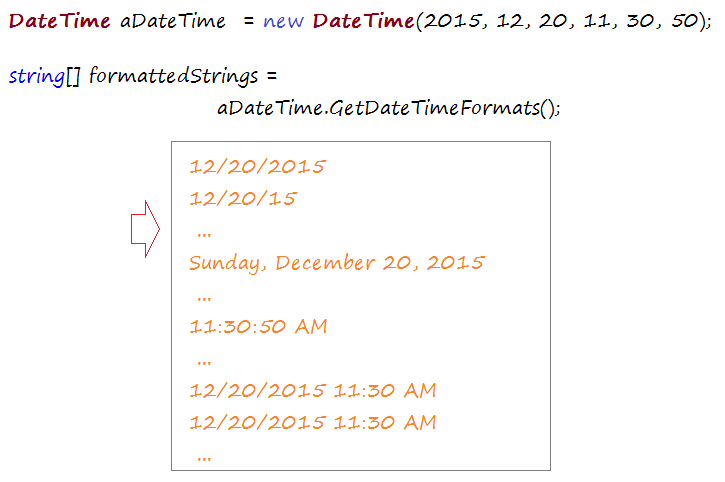
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class AllStandardFormatsDemo
{
public static void Main(string[] args)
{
DateTime aDateTime = new DateTime(2015, 12, 20, 11, 30, 50);
// Поддеживаемые форматы date-time.
string[] formattedStrings = aDateTime.GetDateTimeFormats();
foreach (string format in formattedStrings)
{
Console.WriteLine(format);
}
Console.Read();
}
}
}
12/20/2015
12/20/15
12/20/15
12/20/2015
15/12/20
2015-12-20
20-Dec-15
Sunday, December 20, 2015
December 20, 2015
Sunday, 20 December, 2015
20 December, 2015
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30
Sunday, December 20, 2015 11:30
December 20, 2015 11:30 AM
December 20, 2015 11:30 AM
December 20, 2015 11:30
December 20, 2015 11:30
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30
Sunday, 20 December, 2015 11:30
20 December, 2015 11:30 AM
20 December, 2015 11:30 AM
...
Метод | Описание |
ToString(String, IFormatProvider) | Конвертирует значения текущего объекта DateTime в эквивалентный представляющий string, используя формат данный параметром String, и информацию формата культуры (Culture) данная параметром IFormatProvider. |
ToString(IFormatProvider) | Конвертирует значения текущего объекта DateTime в эквивалентный string с информацией формата культуры (Culture) данный параметром IFormatProvider. |
ToString(String) | Конвертирует значения текущего объекта DateTime в эквивалентный string используя формат данный параметром String и конвенции форматирования текущей культуры. |
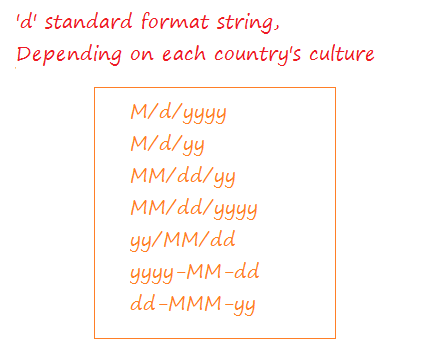
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Globalization;
namespace DateTimeTutorial
{
class SimpleDateTimeFormat
{
public static void Main(string[] args)
{
DateTime aDateTime = new DateTime(2015, 12, 20, 11, 30, 50);
Console.WriteLine("DateTime: " + aDateTime);
String d_formatString = aDateTime.ToString("d");
Console.WriteLine("Format 'd' : " + d_formatString);
// Объект описывает культуру Америки (en-US Culture)
CultureInfo enUs = new CultureInfo("en-US");
// ==> 12/20/2015 (MM/dd/yyyy)
Console.WriteLine("Format 'd' & en-US: " + aDateTime.ToString("d", enUs));
// По культуре Вьетнама.
CultureInfo viVn = new CultureInfo("vi-VN");
// ==> 12/20/2015 (dd/MM/yyyy)
Console.WriteLine("Format 'd' & vi-VN: " + aDateTime.ToString("d", viVn));
Console.Read();
}
}
}
DateTime: 12/20/2015 11:30:50 AM
Format 'd' : 12/20/2015
Format 'd' & en-US: 12/20/2015
Format 'd' & vi-VN: 20/12/2015
Код (Code) | Образец (Pattern) |
"d" | Короткая дата |
"D" | Длинная дата |
"f" | Короткая дата, короткое время |
"F" | Длинная дата, длинное время |
"g" | Общая дата время. Короткое время. |
"G" | Общая дата время. Длинное время. |
"M", 'm" | Месяц/день. |
"O", "o" | Дата/время поездки. |
"R", "r" | RFC1123 |
"s" | Сортированная дата время |
"t" | Короткое время |
"T" | Длинное время |
"u" | Универсальное сортируемое время дата (Universal sortable date time). |
"U" | Полное универсальное сортируемое время дата (Universal full date time). |
"Y", "y" | Год месяц |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class SimpleDateTimeFormatAll
{
public static void Main(string[] args)
{
char[] formats = {'d', 'D','f','F','g','G','M', 'm','O', 'o','R', 'r','s','t','T','u','U','Y', 'y'};
DateTime aDateTime = new DateTime(2015, 12, 20, 11, 30, 50);
foreach (char ch in formats)
{
Console.WriteLine("\n======" + ch + " ========\n");
// Поддерживаемые форматы date-time.
string[] formattedStrings = aDateTime.GetDateTimeFormats(ch);
foreach (string format in formattedStrings)
{
Console.WriteLine(format);
}
}
Console.ReadLine();
}
}
}
======d ========
12/20/2015
12/20/15
12/20/15
12/20/2015
15/12/20
2015-12-20
20-Dec-15
======D ========
Sunday, December 20, 2015
December 20, 2015
Sunday, 20 December, 2015
20 December, 2015
======f ========
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30
Sunday, December 20, 2015 11:30
December 20, 2015 11:30 AM
December 20, 2015 11:30 AM
December 20, 2015 11:30
December 20, 2015 11:30
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30
Sunday, 20 December, 2015 11:30
20 December, 2015 11:30 AM
20 December, 2015 11:30 AM
20 December, 2015 11:30
20 December, 2015 11:30
======F ========
Sunday, December 20, 2015 11:30:50 AM
Sunday, December 20, 2015 11:30:50 AM
Sunday, December 20, 2015 11:30:50
Sunday, December 20, 2015 11:30:50
December 20, 2015 11:30:50 AM
December 20, 2015 11:30:50 AM
December 20, 2015 11:30:50
December 20, 2015 11:30:50
Sunday, 20 December, 2015 11:30:50 AM
Sunday, 20 December, 2015 11:30:50 AM
Sunday, 20 December, 2015 11:30:50
Sunday, 20 December, 2015 11:30:50
20 December, 2015 11:30:50 AM
20 December, 2015 11:30:50 AM
20 December, 2015 11:30:50
20 December, 2015 11:30:50
======g ========
12/20/2015 11:30 AM
12/20/2015 11:30 AM
12/20/2015 11:30
12/20/2015 11:30
12/20/15 11:30 AM
12/20/15 11:30 AM
12/20/15 11:30
12/20/15 11:30
12/20/15 11:30 AM
12/20/15 11:30 AM
12/20/15 11:30
12/20/15 11:30
12/20/2015 11:30 AM
12/20/2015 11:30 AM
12/20/2015 11:30
12/20/2015 11:30
15/12/20 11:30 AM
15/12/20 11:30 AM
15/12/20 11:30
15/12/20 11:30
2015-12-20 11:30 AM
2015-12-20 11:30 AM
2015-12-20 11:30
2015-12-20 11:30
20-Dec-15 11:30 AM
20-Dec-15 11:30 AM
20-Dec-15 11:30
20-Dec-15 11:30
======G ========
12/20/2015 11:30:50 AM
12/20/2015 11:30:50 AM
12/20/2015 11:30:50
12/20/2015 11:30:50
12/20/15 11:30:50 AM
12/20/15 11:30:50 AM
12/20/15 11:30:50
12/20/15 11:30:50
12/20/15 11:30:50 AM
12/20/15 11:30:50 AM
12/20/15 11:30:50
12/20/15 11:30:50
12/20/2015 11:30:50 AM
12/20/2015 11:30:50 AM
12/20/2015 11:30:50
12/20/2015 11:30:50
15/12/20 11:30:50 AM
15/12/20 11:30:50 AM
15/12/20 11:30:50
15/12/20 11:30:50
2015-12-20 11:30:50 AM
2015-12-20 11:30:50 AM
2015-12-20 11:30:50
2015-12-20 11:30:50
20-Dec-15 11:30:50 AM
20-Dec-15 11:30:50 AM
20-Dec-15 11:30:50
20-Dec-15 11:30:50
======M ========
December 20
======m ========
December 20
======O ========
2015-12-20T11:30:50.0000000
======o ========
2015-12-20T11:30:50.0000000
======R ========
Sun, 20 Dec 2015 11:30:50 GMT
======r ========
Sun, 20 Dec 2015 11:30:50 GMT
======s ========
2015-12-20T11:30:50
======t ========
11:30 AM
11:30 AM
11:30
11:30
======T ========
11:30:50 AM
11:30:50 AM
11:30:50
11:30:50
======u ========
2015-12-20 11:30:50Z
======U ========
Sunday, December 20, 2015 4:30:50 AM
Sunday, December 20, 2015 04:30:50 AM
Sunday, December 20, 2015 4:30:50
Sunday, December 20, 2015 04:30:50
December 20, 2015 4:30:50 AM
December 20, 2015 04:30:50 AM
December 20, 2015 4:30:50
December 20, 2015 04:30:50
Sunday, 20 December, 2015 4:30:50 AM
Sunday, 20 December, 2015 04:30:50 AM
Sunday, 20 December, 2015 4:30:50
Sunday, 20 December, 2015 04:30:50
20 December, 2015 4:30:50 AM
20 December, 2015 04:30:50 AM
20 December, 2015 4:30:50
20 December, 2015 04:30:50
======Y ========
December, 2015
======y ========
December, 2015
// Время, которое вы видите на Http Header
string httpTime = "Fri, 21 Feb 2011 03:11:31 GMT";
// Время, которое вы видите на w3.org
string w3Time = "2016/05/26 14:37:11";
// Время, которое вы видите на nytimes.com
string nyTime = "Thursday, February 26, 2012";
// Время по стандарту ISO 8601.
string isoTime = "2016-02-10";
// Время при просмотре даты времени созданное/отредактированное на Windows.
string windowsTime = "11/21/2015 11:35 PM";
// Время на "Windows Date and Time panel"
string windowsPanelTime = "11:07:03 PM";
7. Кастомизировать форматs DateTime
string format = "dd/MM/yyyy HH:mm:ss";
DateTime now = DateTime.Now;
// ==> 18/03/2016 23:49:39
string s = now.ToString(format);
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class CustomDateTimeFormatExample
{
public static void Main(string[] args)
{
// Формат даты и времени.
string format = "dd/MM/yyyy HH:mm:ss";
DateTime now = DateTime.Now;
string s = now.ToString(format);
Console.WriteLine("Now: " + s);
Console.Read();
}
}
}
public static DateTime Parse(string s)
public static DateTime Parse(string s, IFormatProvider provider)
public static DateTime Parse(string s, IFormatProvider provider, DateTimeStyles styles)
public static bool TryParseExact(string s, string format,
IFormatProvider provider, DateTimeStyles style,
out DateTime result)
public static bool TryParseExact(string s, string[] formats,
IFormatProvider provider, DateTimeStyles style,
out DateTime result)
Method | Example |
static DateTime Parse(string) | // See also Standard DateTime format. string httpHeaderTime = "Fri, 21 Feb 2011 03:11:31 GMT"; DateTime.Parse(httpHeaderTime); |
static DateTime ParseExact(
string s, string format, IFormatProvider provider ) | string dateString = "20160319 09:57";
DateTime.ParseExact(dateString ,"yyyyMMdd HH:mm",null); |
static bool TryParseExact(
string s, string format, IFormatProvider provider, DateTimeStyles style, out DateTime result ) | This method is very similar to ParseExact, but it returns a boolean, true if the time series is parsed, otherwise it returns false.
(See example below). |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class ParseDateStringExample
{
public static void Main(string[] args)
{
string dateString = "20160319 09:57";
// Использовать ParseExact для парсинга String в DateTime.
DateTime dateTime = DateTime.ParseExact(dateString, "yyyyMMdd HH:mm", null);
Console.WriteLine("Input dateString: " + dateString);
Console.WriteLine("Parse Results: " + dateTime.ToString("dd-MM-yyyy HH:mm:ss"));
// Строка datetime имеет пробел впереди.
dateString = " 20110319 11:57";
// Использовать метод TryParseExact.
// Данный метод возвращает true если строка 'dateString' может быть парсирована.
// И возвращает значение параметру 'out dateTime'.
bool successful = DateTime.TryParseExact(dateString, "yyyyMMdd HH:mm", null,
System.Globalization.DateTimeStyles.AllowLeadingWhite,
out dateTime);
Console.WriteLine("\n------------------------\n");
Console.WriteLine("Input dateString: " + dateString);
Console.WriteLine("Can Parse? :" + successful);
if (successful)
{
Console.WriteLine("Parse Results: " + dateTime.ToString("dd-MM-yyyy HH:mm:ss"));
}
Console.Read();
}
}
}
Input dateString: 20160319 09:57
Parse Results: 19-0302016 09:57:00
------------------------
Input dateString: 20110319 11:57
Can Parse? :True
Parse Results: 19-03-2011 11:57:00
Pуководства C#
- Наследование и полиморфизм в C#
- Что мне нужно для начала работы с C#?
- Быстрый обучение C# для начинающих
- Установите Visual Studio 2013 в Windows
- Абстрактный класс и Interface в C#
- Установите Visual Studio 2015 в Windows
- Сжатие и декомпрессия в C#
- Руководство по программированию многопоточности C#
- Руководство C# Delegate и Event
- Установите AnkhSVN в Windows
- Программирование C# для группы использующей Visual Studio и SVN
- Установить .Net Framework
- Access Modifier (Модификатор доступа) в C#
- Руководство C# String и StringBuilder
- Руководство C# Property
- Руководство C# Enum
- Руководство C# Structure
- Руководство C# Generics
- Обработка исключений для C#
- Руководство C# Date Time
- Манипулирование файлами и каталогами в C#
- Руководство CSharp Streams - двоичные потоки в C#
- Руководство Регулярное выражение C#
- Подключиться к базе данных SQL Server в C#
- Работа с базой данных SQL Server на C#
- Подключиться к базе данных MySQL в C#
- Работа с базой данных MySQL на C#
- Подключиться к базе данных Oracle в C# без Oracle Client
- Работа с базой данных Oracle на C#