Создайте Java RESTful Client с помощью Jersey Client
1. Цель статьи
В данной статье я покажу вам как создать приложение Java RESTful Client используя Jersey Client API чтобы вызвать RESTful web service.
Примеры в данной статье ссылаются на RESTful Web service в предыдущем руководстве, можете посмотреть по данной ссылке:
2. Создать Maven Project
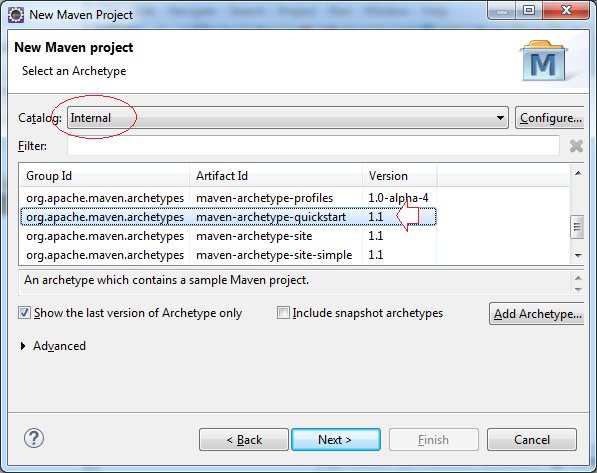
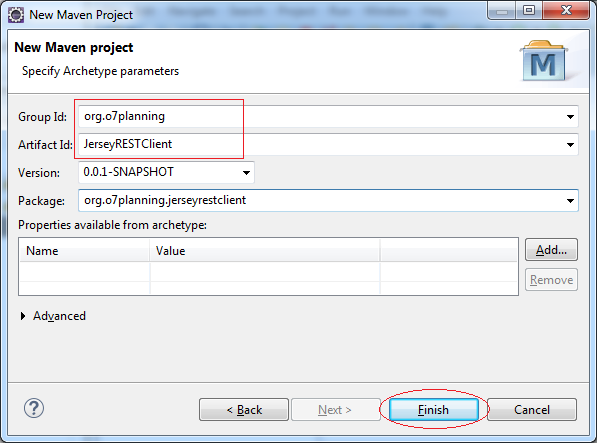
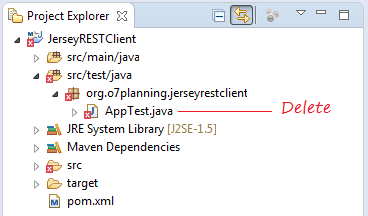
Удостоверьтесь что ваш project использует Java >=6, потому что в данном project используется библиотека JAXB, имеющаяся в Jdk6 или более обновленный.
Project Properties:
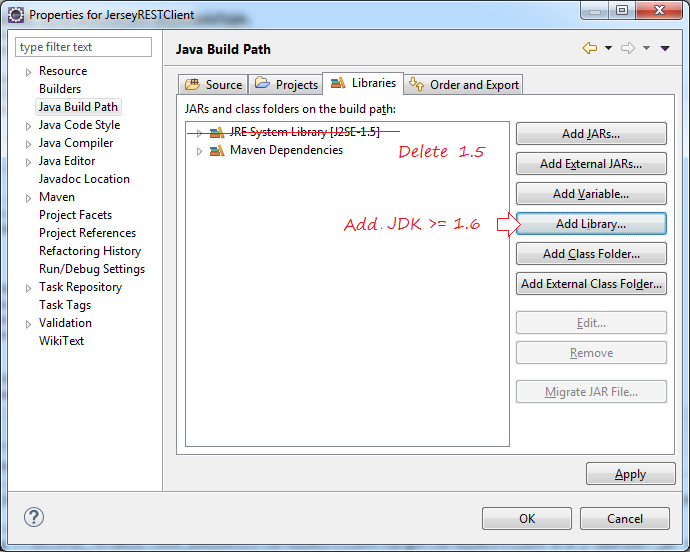
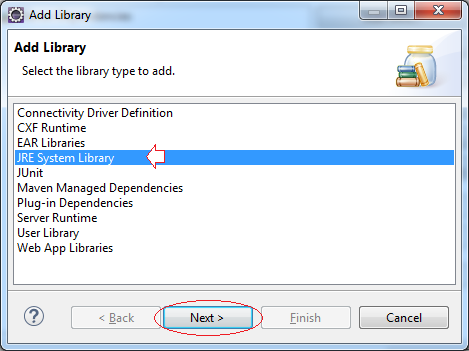
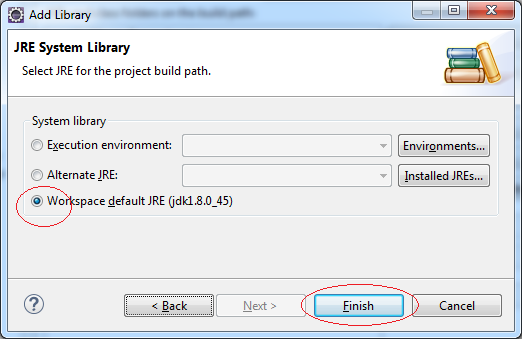
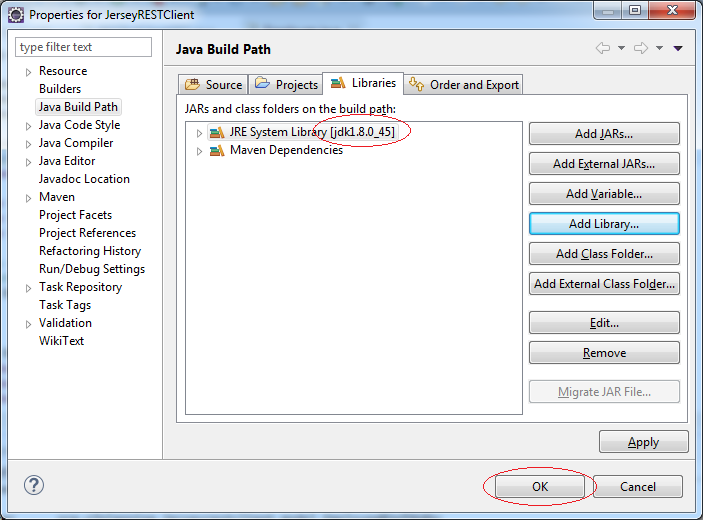
Объявить библиотеку Jersey Client:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>JerseyRESTClient</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>JerseyRESTClient</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.jersey/jersey-client -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-client</artifactId>
<version>1.19.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.jersey/jersey-json -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-json</artifactId>
<version>1.19.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.jersey/jersey-bundle -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-bundle</artifactId>
<version>1.19.2</version>
</dependency>
</dependencies>
</project>
3. Model Classes
По умолчанию Jersey API использует JAXB это XML-Binding по умолчанию, использующийся для конвертации объектов Java в XML и обратно, вы прикрепляете Annotation в JAXB к классам model для аннотирования конвертации для JAXB.
И MOXy является JSON-Binding по умолчанию, чтобы Jersey конвертировал объекты JSON в Java и обратно, вам не нужно прикреплять Annotation к классам model.
И MOXy является JSON-Binding по умолчанию, чтобы Jersey конвертировал объекты JSON в Java и обратно, вам не нужно прикреплять Annotation к классам model.
Employee.java
package org.o7planning.jerseyrestclient.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "employee")
@XmlAccessorType(XmlAccessType.FIELD)
public class Employee {
private String empNo;
private String empName;
private String position;
// This default constructor is required if there are other constructors.
public Employee() {
}
public Employee(String empNo, String empName, String position) {
this.empNo = empNo;
this.empName = empName;
this.position = position;
}
public String getEmpNo() {
return empNo;
}
public void setEmpNo(String empNo) {
this.empNo = empNo;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
}
4. Get JSON в String
Пример ниже создает класс, который вызывает RESTful Web Serivce чтобы получить данные в виде JSON, результат возвращает String.
GetJsonAsString.java
package org.o7planning.jerseyrestclient;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
public class GetJsonAsString {
public static void main(String[] args) {
// Create Client
Client client = Client.create();
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees/E01");
ClientResponse response = webResource.accept("application/json").get(ClientResponse.class);
// Status 200 is successful.
if (response.getStatus() != 200) {
System.out.println("Failed with HTTP Error code: " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
String output = response.getEntity(String.class);
System.out.println("Output from Server .... \n");
System.out.println(output);
}
}
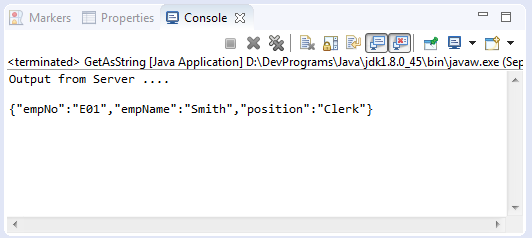
5. Get JSON в Object
В примере ниже Jersey Client конвертирует данные JSON в объекты Java.
GetJsonAsObject.java
package org.o7planning.jerseyrestclient;
import javax.ws.rs.core.MediaType;
import org.o7planning.jerseyrestclient.model.Employee;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.WebResource.Builder;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
import com.sun.jersey.api.json.JSONConfiguration;
public class GetJsonAsObject {
public static void main(String[] args) {
ClientConfig clientConfig = new DefaultClientConfig();
// Create Client based on Config
Client client = Client.create(clientConfig);
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees/E01");
Builder builder = webResource.accept(MediaType.APPLICATION_JSON) //
.header("content-type", MediaType.APPLICATION_JSON);
ClientResponse response = builder.get(ClientResponse.class);
// Status 200 is successful.
if (response.getStatus() != 200) {
System.out.println("Failed with HTTP Error code: " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
System.out.println("Output from Server .... \n");
Employee employee = (Employee) response.getEntity(Employee.class);
System.out.println("Emp No .... " + employee.getEmpNo());
System.out.println("Emp Name .... " + employee.getEmpName());
System.out.println("Position .... " + employee.getPosition());
}
}
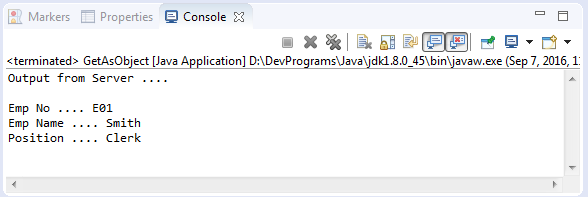
6. Get JSON в List
Jersey может конвертировать JSON в List.
Например, RESTful Web Service возвращает List объектов.
@Path("/employees")
public class EmployeeService {
// URI:
// /contextPath/servletPath/employees
@GET
@Produces({ MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML })
public List<Employee> getEmployees() {
List<Employee> listOfCountries = EmployeeDAO.getAllEmployees();
return listOfCountries;
}
}
Пример Client:
GetJsonAsList.java
package org.o7planning.jerseyrestclient;
import java.util.List;
import javax.ws.rs.core.MediaType;
import org.o7planning.jerseyrestclient.model.Employee;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.GenericType;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.WebResource.Builder;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
public class GetJsonAsList {
public static void main(String[] args) {
ClientConfig clientConfig = new DefaultClientConfig();
// Create Client based on Config
Client client = Client.create(clientConfig);
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees");
Builder builder = webResource.accept(MediaType.APPLICATION_JSON) //
.header("content-type", MediaType.APPLICATION_JSON);
ClientResponse response = builder.get(ClientResponse.class);
// Status 200 is successful.
if (response.getStatus() != 200) {
System.out.println("Failed with HTTP Error code: " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
GenericType<List<Employee>> generic = new GenericType<List<Employee>>() {
// No thing
};
List<Employee> list = response.getEntity(generic);
System.out.println("Output from Server .... \n");
for (Employee emp : list) {
System.out.println(" --- ");
System.out.println("Emp No .... " + emp.getEmpNo());
System.out.println("Emp Name .... " + emp.getEmpName());
System.out.println("Position .... " + emp.getPosition());
}
}
}
Запуск примера:
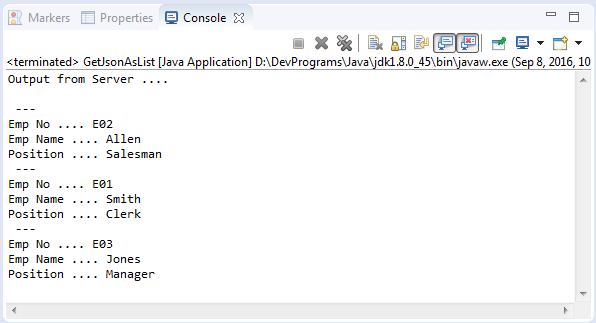
7. Get XML в String
GetXmlAsString.java
package org.o7planning.jerseyrestclient;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
public class GetXmlAsString {
public static void main(String[] args) {
// Create Client
Client client = Client.create();
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees/E01");
ClientResponse response = webResource.accept("application/xml").get(ClientResponse.class);
// Status 200 is successful.
if (response.getStatus() != 200) {
System.out.println("Failed with HTTP Error code: " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
String output = response.getEntity(String.class);
System.out.println("Output from Server .... \n");
System.out.println(output);
}
}
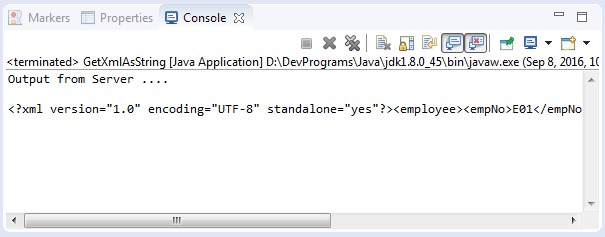
8. Get XML в Object
GetXmlAsObject.java
package org.o7planning.jerseyrestclient;
import javax.ws.rs.core.MediaType;
import org.o7planning.jerseyrestclient.model.Employee;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.WebResource.Builder;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
public class GetXmlAsObject {
public static void main(String[] args) {
ClientConfig clientConfig = new DefaultClientConfig();
// Create Client based on Config
Client client = Client.create(clientConfig);
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees/E01");
Builder builder = webResource.accept(MediaType.APPLICATION_XML) //
.header("content-type", MediaType.APPLICATION_XML);
ClientResponse response = builder.get(ClientResponse.class);
// Status 200 is successful.
if (response.getStatus() != 200) {
System.out.println("Failed with HTTP Error code: " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
System.out.println("Output from Server .... \n");
Employee employee = (Employee) response.getEntity(Employee.class);
System.out.println("Emp No .... " + employee.getEmpNo());
System.out.println("Emp Name .... " + employee.getEmpName());
System.out.println("Position .... " + employee.getPosition());
}
}
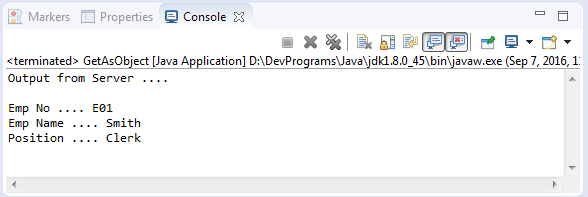
9. Get XML в List
Пример RESTful Web Service возвращает List объектов.
@Path("/employees")
public class EmployeeService {
// URI:
// /contextPath/servletPath/employees
@GET
@Produces({ MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML })
public List<Employee> getEmployees() {
List<Employee> listOfCountries = EmployeeDAO.getAllEmployees();
return listOfCountries;
}
}
GetXmlAsList.java
package org.o7planning.jerseyrestclient;
import java.util.List;
import javax.ws.rs.core.MediaType;
import org.o7planning.jerseyrestclient.model.Employee;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.GenericType;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.WebResource.Builder;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
public class GetXmlAsList {
public static void main(String[] args) {
ClientConfig clientConfig = new DefaultClientConfig();
// Create Client based on Config
Client client = Client.create(clientConfig);
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees");
Builder builder = webResource.accept(MediaType.APPLICATION_XML) //
.header("content-type", MediaType.APPLICATION_XML);
ClientResponse response = builder.get(ClientResponse.class);
// Status 200 is successful.
if (response.getStatus() != 200) {
System.out.println("Failed with HTTP Error code: " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
GenericType<List<Employee>> generic = new GenericType<List<Employee>>() {
// No thing
};
List<Employee> list = response.getEntity(generic);
System.out.println("Output from Server .... \n");
for (Employee emp : list) {
System.out.println(" --- ");
System.out.println("Emp No .... " + emp.getEmpNo());
System.out.println("Emp Name .... " + emp.getEmpName());
System.out.println("Position .... " + emp.getPosition());
}
}
}
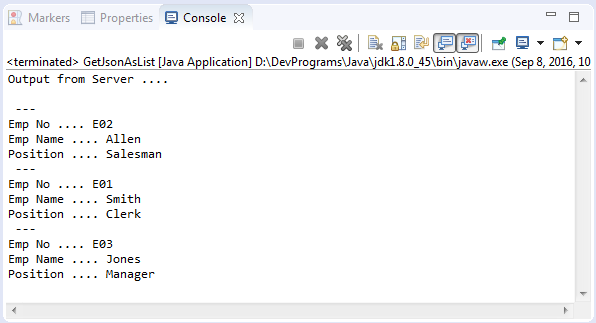
10. Post JSON
Используя POST сделать запрос Web serivce чтобы создать новый рес. В примере ниже создается запрос POST отправленный с данными JSON чтобы сделать запрос создать новый Employee.
PostJsonString.java
package org.o7planning.jerseyrestclient;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
public class PostJsonString {
public static void main(String[] args) {
Client client = Client.create();
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees");
// Data send to web service.
String input = "{\"empNo\":\"E01\",\"empName\":\"New Emp1\",\"position\":\"Manager\"}";
ClientResponse response = webResource.type("application/json").post(ClientResponse.class, input);
if (response.getStatus() != 200) {
System.out.println("Failed : HTTP error code : " + response.getStatus());
String error= response.getEntity(String.class);
System.out.println("Error: "+error);
return;
}
System.out.println("Output from Server .... \n");
String output = response.getEntity(String.class);
System.out.println(output);
}
}
Запуск примера:
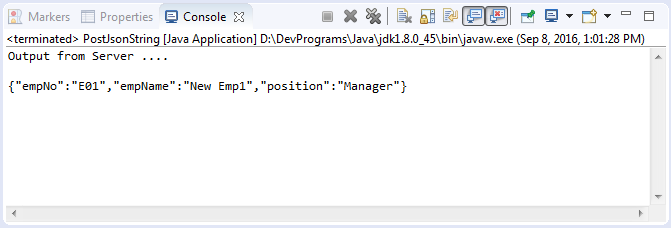
Jersey Client может автоматически конвертировать объект Java в JSON или XML чтобы отправить с запросом.
PostJsonObject.java
package org.o7planning.jerseyrestclient;
import org.o7planning.jerseyrestclient.model.Employee;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
public class PostJsonObject {
public static void main(String[] args) {
Client client = Client.create();
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees");
// This object will be automatically converted into JSON
Employee newEmp = new Employee("E05", "New Emp1", "Manager");
ClientResponse response = webResource.type("application/json").post(ClientResponse.class, newEmp);
if (response.getStatus() != 200) {
System.out.println("Failed : HTTP error code : " + response.getStatus());
String error = response.getEntity(String.class);
System.out.println("Error: " + error);
return;
}
System.out.println("Output from Server .... \n");
String output = response.getEntity(String.class);
System.out.println(output);
}
}
11. Post XML
PostXmlString.java
package org.o7planning.jerseyrestclient;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
public class PostXmlString {
public static void main(String[] args) {
Client client = Client.create();
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees");
// Data send to web service.
String input = "<employee>"//
+ "<empNo>E05</empNo>"//
+ "<empName>New Emp1</empName>"//
+ "<position>Manager</position>"
+ "</employee>";
// Send XML and receive XML
ClientResponse response = webResource.type("application/xml")//
.accept("application/xml")//
.post(ClientResponse.class, input);
if (response.getStatus() != 200) {
System.out.println("Failed : HTTP error code : " + response.getStatus());
String error = response.getEntity(String.class);
System.out.println("Error: " + error);
return;
}
System.out.println("Output from Server .... \n");
String output = response.getEntity(String.class);
System.out.println(output);
}
}
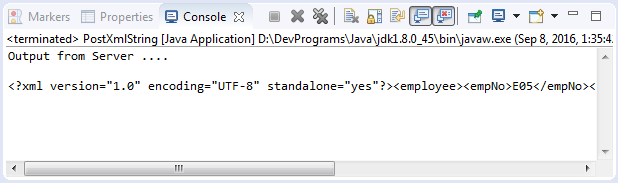
Jersey Client может автоматичкски конвертировать объект Java в JSON или XML чтобы отправить с запросом.
PostXmlObject.java
package org.o7planning.jerseyrestclient;
import org.o7planning.jerseyrestclient.model.Employee;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
public class PostXmlObject {
public static void main(String[] args) {
Client client = Client.create();
WebResource webResource = client.resource("http://localhost:8080/RESTfulCRUD/rest/employees");
// This object will be automatically converted into XML
Employee newEmp = new Employee("E05", "New Emp1", "Manager");
// Send XML and receive XML
ClientResponse response = webResource.type("application/xml")//
.accept("application/xml") //
.post(ClientResponse.class, newEmp);
if (response.getStatus() != 200) {
System.out.println("Failed : HTTP error code : " + response.getStatus());
String error = response.getEntity(String.class);
System.out.println("Error: " + error);
return;
}
System.out.println("Output from Server .... \n");
String output = response.getEntity(String.class);
System.out.println(output);
}
}
Руководства Java Web Services
- Что такое RESTful Web Service?
- Руководство Java RESTful Web Services для начинающих
- Простой пример CRUD с Java RESTful Web Service
- Создайте Java RESTful Client с помощью Jersey Client
- Отладчик RESTClient для RESTful WebServices
- Простой пример CRUD с Spring MVC RESTful Web Service
- Пример CRUD Restful Web Service c Spring Boot
- Пример Spring Boot Restful Client c RestTemplate
- Защита Spring Boot RESTful Service используя Basic Authentication
Show More