Руководство AngularJS Filter
1. AngularJS Filters
В AngularJS, Filter (Фильтр) используется для форматирования данных, отображенных для пользователей.
Ниже является общий синтаксис использующий Filter:
Filters Usage
{{ expression [| filter_name[:parameter_value] ... ] }}
AngularJS построил некоторые готовые основные Filter, и вы можете их использовать.
Filter | Description |
lowercase | Format a string to lower case. |
uppercase | Format a string to upper case. |
number | Format a number to a string. |
currency | Format a number to a currency format. |
date | Format a date to a specified format. |
json | Format an object to a JSON string. |
filter | Select a subset of items from an array. |
limitTo | Limits an array/string, into a specified number of elements/characters. |
orderBy | Orders an array by an expression. |
2. uppercase/lowercase
upperlowercase-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.greeting = "Hello World";
});
upperlowercase-example
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="upperlowercase-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filters: uppercase/lowercase</h3>
<p>Origin String: {{ greeting }}</p>
<p>Uppercase: {{ greeting | uppercase }}</p>
<p>Lowercase: {{ greeting | lowercase }}</p>
</div>
</body>
</html>
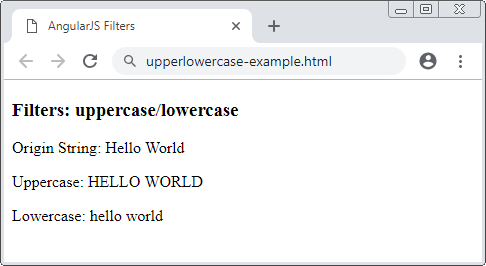
3. number
Фильтр (filter) number помогает форматировать число в строку.
Способ использования в HTML:
<!-- fractionSize: Optional -->
{{ number_expression | number : fractionSize }}
Способ использования в Javascript:
// fractionSize: Optional
$filter('number')( aNumber , fractionSize )
Параметры:
aNumber |
number
string | Число для форматирования. |
fractionSize (Optional) | number
string | Количество позиций для десятичной части (decimal). Если вы не предоставляете значение данному параметру, его значение зависит от locale. В случае locale по умолчанию, его значение будет 3. |
number-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="number-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: number</h3>
<p><b>Origin Number:</b> {{ revenueAmount }}</p>
<p><b>Default Number Format:</b> {{ revenueAmount | number }}</p>
<p><b>Format with 2 decimal places:</b> {{ revenueAmount | number : 2 }}</p>
</div>
</body>
</html>
number-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.revenueAmount = 20011.2345;
});
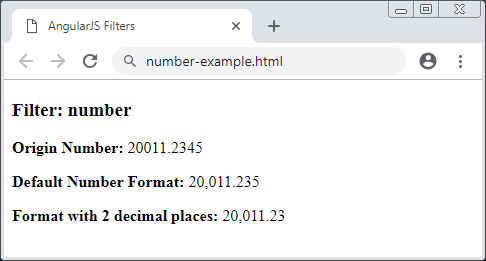
Пример использования фильтра number в Javascript:
number-example2.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope, $filter) {
var aNumber = 19001.2345;
// Use number filter in Javascript:
$scope.revenueAmountStr = $filter("number")(aNumber, 2);// ==> 19,001.23
});
4. currency
Фильтр (filter) currency используется для форматирования числа в валюту (Например $1,234.56), в основном вам нужно предоставить сивол (symbol) валюты для форматирования, или же будет использован настоящий символ валюты по умолчанию у locale.
Способ использования в HTML:
{{ amount | currency : symbol : fractionSize}}
Способ использования в Javascript:
$filter('currency')(amount, symbol, fractionSize)
Параметры:
amount | number | Число. |
symbol (необязательно) | string | Символ (symbol) валюты, или эквивалентно для отображения. |
fractionSize (необязательно) | number | Количество позиций для десятичной части (decimal). Если не будет предоставлено, значение будет по настоящему locale по умолчанию. |
Пример:
currency-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="currency-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
Обычно сивол валюты будет стоять перед суммой (Например €123.45), это зависит от locale. Вы можете определить locale который вы хотите использовать, например locale_de-de, тогда вы получите результат 123.45€.
Поиск библиотек locale у AngularJS:
currency-locale-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-locale-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<!-- Use locale_de-de -->
<!-- https://cdnjs.com/libraries/angular-i18n -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular-i18n/1.7.4/angular-locale_de-de.js"></script>
<script src="currency-locale-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency (LOCALE: de-de)</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
5. date
Фильтр date используется для форматирования объекта Date в строку.
Способ использования фильтра date в HTML:
{{ date_expression | aDate : format : timezone}}
Способ использования фильтра date в Javascript:
$filter('date')(aDate, format, timezone)
Параметры:
TODO
Пример:
date-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myDate = new Date();
});
date-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="date-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: date</h3>
<p>
<!-- $scope.myDate = new Date() -->
<b ng-non-bindable>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</b>:
<span>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'medium'}}</b>:
<span>{{1288323623006 | date:'medium'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</b>:
<span>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'MM/dd/yyyy @ h:mma'}}</b>:
<span>{{'1288323623006' | date:'MM/dd/yyyy @ h:mma'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:"MM/dd/yyyy 'at' h:mma"}}</b>:
<span>{{'1288323623006' | date:"MM/dd/yyyy 'at' h:mma"}}</span>
</p>
</div>
</body>
</html>
6. json
Фильтр json используется для конвертирования объекта (object) в строку формата JSON.
Способ использования в HTML:
{{ json_expression | json : spacing}}
Способ использования в Javascript:
$filter('json')(object, spacing)
Параметры:
object | * | Любой объект JavaScript (включая массивы и примитивные виды (primitive) ) |
spacing (optional) | number | Количество пробелов, использующиеся для отступа (indentation), по умолчанию 2. |
Пример:
json-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.department = {
id : 1,
name: "Sales",
employees : [
{id: 1, fullName: "Tom", gender: "Male"},
{id: 2, fullName: "Jerry", gender: "Male"}
]
};
});
json-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="json-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: json</h3>
<p>
<b ng-non-bindable>{{ department | json : 5 }}</b>:
<pre>{{ department | json : 5}}</pre>
</p>
<p>
<b ng-non-bindable>{{ department | json }}</b>:
<pre>{{ department | json }}</pre>
</p>
</div>
</body>
</html>
7. limitTo
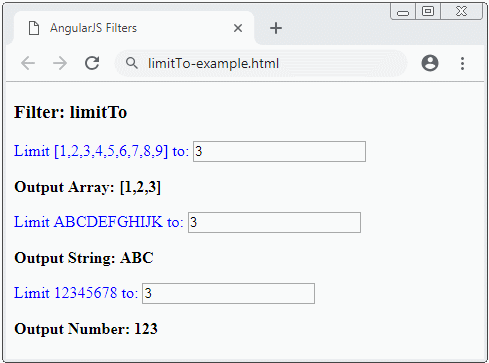
Способ использования в HTML:
{{ input | limitTo : limit : begin}}
Способ использования в Javascript:
$filter('limitTo')(input, limitLength, startIndex)
Параметры:
Пример:
limitTo-example.js
var app = angular.module("myApp", [ ]);
app.controller("myCtrl", function($scope) {
$scope.anArray = [1,2,3,4,5,6,7,8,9];
$scope.aString = "ABCDEFGHIJK";
$scope.aNumber = 12345678;
$scope.arrayLimit = 3;
$scope.stringLimit = 3;
$scope.numberLimit = 3;
});
limitTo-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="limitTo-example.js"></script>
<style>
p {color: blue;}
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: limitTo</h3>
<p>
Limit {{anArray}} to:
<input type="number" step="1" ng-model="arrayLimit">
</p>
<b>Output Array: {{ anArray | limitTo: arrayLimit }}</b>
<p>
Limit {{aString}} to:
<input type="number" step="1" ng-model="stringLimit">
</p>
<b>Output String: {{ aString | limitTo: stringLimit }}</b>
<p>
Limit {{aNumber}} to:
<input type="number" step="1" ng-model="numberLimit">
</p>
<b>Output Number: {{ aNumber | limitTo: numberLimit }}</b>
</div>
</body>
</html>
8. filter
Фильтр filter используется для выбора поднабора (subset) в массиве и возвращает новый массив.
Способ использования в HTML:
{{ inputArray | filter : expression : comparator : anyPropertyKey}}
Способ использования в Javascript:
$filter('filter')(inputArray, expression, comparator, anyPropertyKey)