Манипулирование файлами и папками в Google Drive с использованием Java
1. Цель статьи
Смотрите так же:
В данной статье я покажу вам как манипулировать с Google Drive через Google Drive Java API. Темы которые будут обсуждать в данной статье включают:
- Создать приложение Java и объявить библиотеки для использования Google Drive API.
- Создать полномочия (Credentials) для взаимодействия с Google Drive.
- Манипулировать с файлами и папками на Google Drive через Google Drive API.
2. Создать Credentials (полномочия)
Например у вас есть аккаунт Gmail это abc@gmail.com, при этом Google предоставить вам бесплатный объем пространства жесткого диска 15GB на Google Drive. Вы можете хранить там свои файлы. Чтобы ваше приложение могло манипулировать с файлами на Google Drive, ему понадобятся Credentials (полномочия). Credentials это просто файл, расположенный на компьютере, где развернуто ваше приложение. Как в изображении ниже:
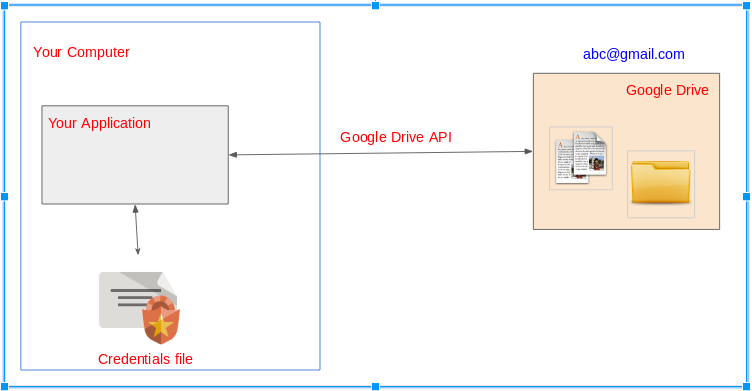
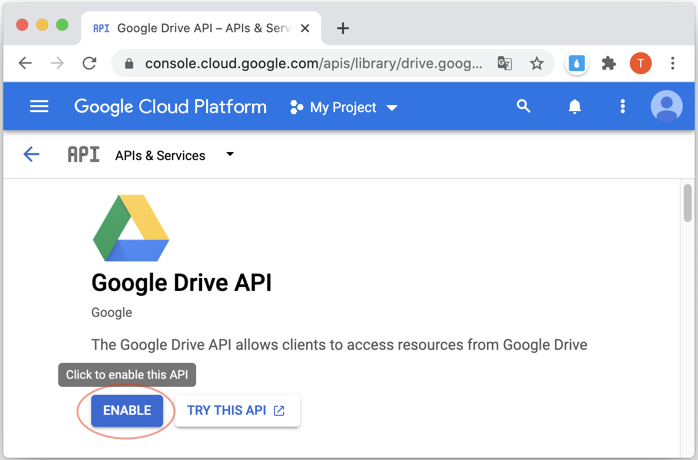
Чтобы получить Credentials (Полномочия) выше, вам нужно создать проект на Google Developer Console, и скачать файл client_secret.json.
3. Java Google Drive API
С проектами использующими Maven, вам нужно объявить следующие зависимые (dependencies):
<!-- https://mvnrepository.com/artifact/com.google.apis/google-api-services-drive -->
<dependency>
<groupId>com.google.apis</groupId>
<artifactId>google-api-services-drive</artifactId>
<version>v3-rev105-1.23.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client</artifactId>
<version>1.23.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.oauth-client/google-oauth-client-jetty -->
<dependency>
<groupId>com.google.oauth-client</groupId>
<artifactId>google-oauth-client-jetty</artifactId>
<version>1.23.0</version>
</dependency>
4. Быстро начать с примером
Создать проект Maven, и создать класс DriveQuickstart чтобы быстро начать с Google Drive API.
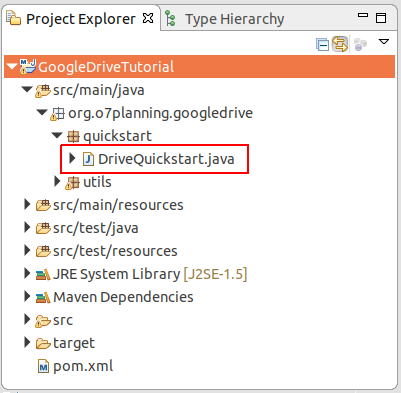
DriveQuickstart.java
package org.o7planning.googledrive.quickstart;
import com.google.api.client.auth.oauth2.Credential;
import com.google.api.client.extensions.java6.auth.oauth2.AuthorizationCodeInstalledApp;
import com.google.api.client.extensions.jetty.auth.oauth2.LocalServerReceiver;
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.http.javanet.NetHttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.jackson2.JacksonFactory;
import com.google.api.client.util.store.FileDataStoreFactory;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.DriveScopes;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.security.GeneralSecurityException;
import java.util.Collections;
import java.util.List;
public class DriveQuickstart {
private static final String APPLICATION_NAME = "Google Drive API Java Quickstart";
private static final JsonFactory JSON_FACTORY = JacksonFactory.getDefaultInstance();
// Directory to store user credentials for this application.
private static final java.io.File CREDENTIALS_FOLDER //
= new java.io.File(System.getProperty("user.home"), "credentials");
private static final String CLIENT_SECRET_FILE_NAME = "client_secret.json";
//
// Global instance of the scopes required by this quickstart. If modifying these
// scopes, delete your previously saved credentials/ folder.
//
private static final List<String> SCOPES = Collections.singletonList(DriveScopes.DRIVE);
private static Credential getCredentials(final NetHttpTransport HTTP_TRANSPORT) throws IOException {
java.io.File clientSecretFilePath = new java.io.File(CREDENTIALS_FOLDER, CLIENT_SECRET_FILE_NAME);
if (!clientSecretFilePath.exists()) {
throw new FileNotFoundException("Please copy " + CLIENT_SECRET_FILE_NAME //
+ " to folder: " + CREDENTIALS_FOLDER.getAbsolutePath());
}
// Load client secrets.
InputStream in = new FileInputStream(clientSecretFilePath);
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in));
// Build flow and trigger user authorization request.
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY,
clientSecrets, SCOPES).setDataStoreFactory(new FileDataStoreFactory(CREDENTIALS_FOLDER))
.setAccessType("offline").build();
return new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user");
}
public static void main(String... args) throws IOException, GeneralSecurityException {
System.out.println("CREDENTIALS_FOLDER: " + CREDENTIALS_FOLDER.getAbsolutePath());
// 1: Create CREDENTIALS_FOLDER
if (!CREDENTIALS_FOLDER.exists()) {
CREDENTIALS_FOLDER.mkdirs();
System.out.println("Created Folder: " + CREDENTIALS_FOLDER.getAbsolutePath());
System.out.println("Copy file " + CLIENT_SECRET_FILE_NAME + " into folder above.. and rerun this class!!");
return;
}
// 2: Build a new authorized API client service.
final NetHttpTransport HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
// 3: Read client_secret.json file & create Credential object.
Credential credential = getCredentials(HTTP_TRANSPORT);
// 5: Create Google Drive Service.
Drive service = new Drive.Builder(HTTP_TRANSPORT, JSON_FACTORY, credential) //
.setApplicationName(APPLICATION_NAME).build();
// Print the names and IDs for up to 10 files.
FileList result = service.files().list().setPageSize(10).setFields("nextPageToken, files(id, name)").execute();
List<File> files = result.getFiles();
if (files == null || files.isEmpty()) {
System.out.println("No files found.");
} else {
System.out.println("Files:");
for (File file : files) {
System.out.printf("%s (%s)\n", file.getName(), file.getId());
}
}
}
}
В первый раз запуска класса DriveQuickstart, создастся папка {user_home}/credentials если она не существует.
Windows | C:\Users\{user}\credentials |
Linux | /home/{user}/credentials |
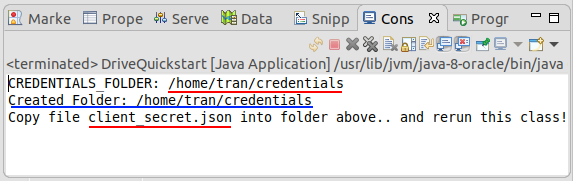
Удостоверьтесь, что у вас есть файл client_secret.json, если нет посмотрите инструкции ниже:
Скопировать client_secret.json в папку, упомянутая выше:
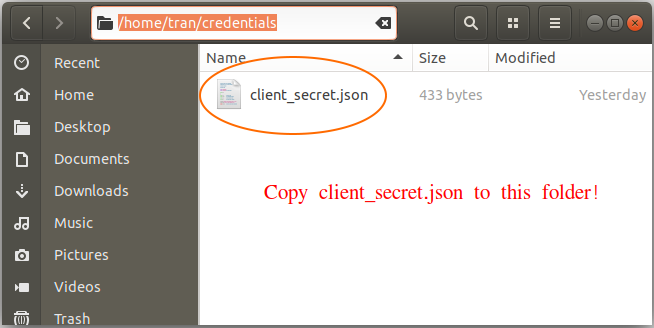
Перезапустить класс DriveQuickstart еще раз. На окне Console скопировать ссылку и пройти по ссылке на браузере.
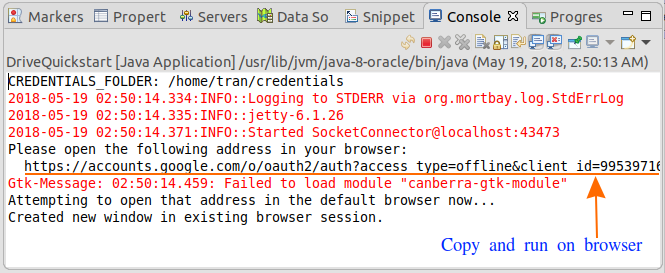
Когда вы заходите в ту ссылку (Link) на браузере, она попросит вас войти в систему с аккаунтом Gmail. Теперь вам нужно войти с аккаунтом Gmail, с которым вы создали файл client_secret.json.
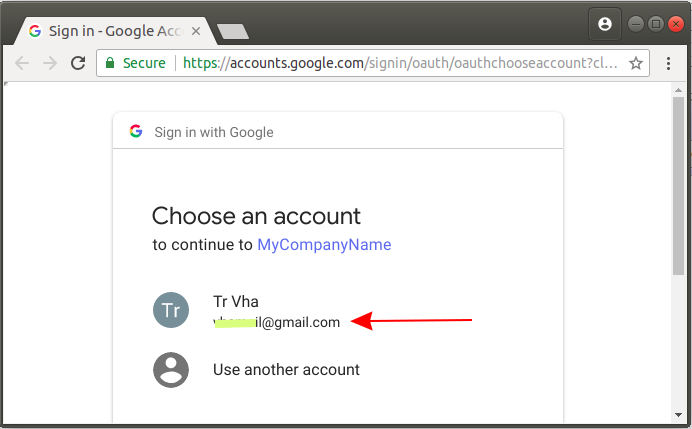
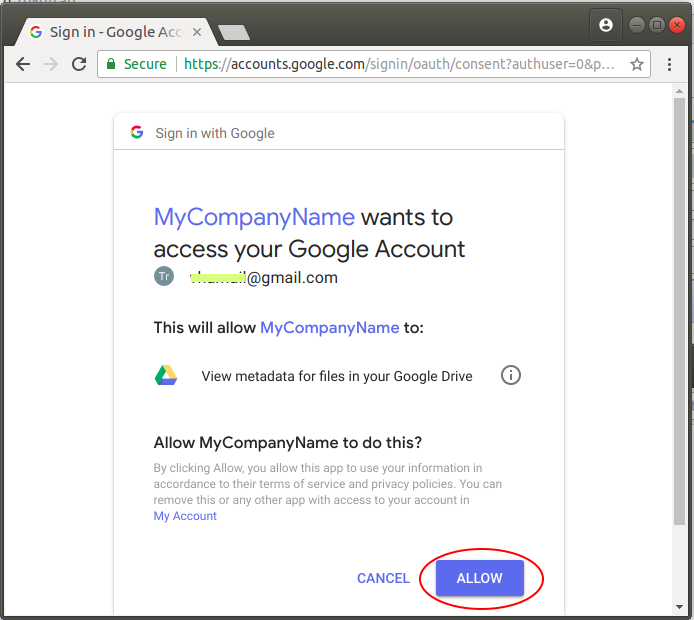
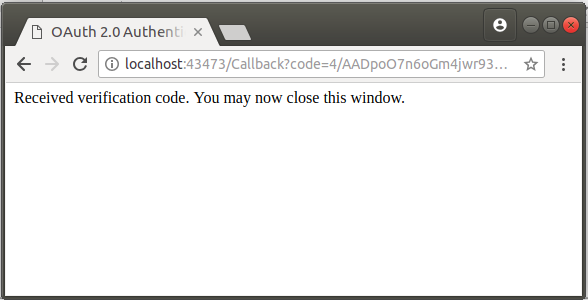
Теперь создастся файл с названием StoredCredential в папке {user_home}/credential.
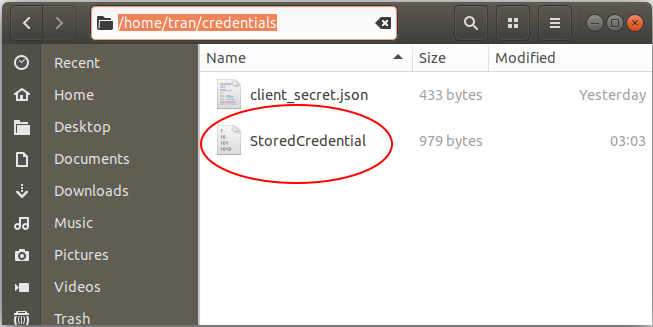
Credential (Полномочия) сохранены на жестком диске вашего компьютера. С данного момента, при работе с Google Drive вам не нужно проходить через шаги выше.
Перезапустить класс DriveQuickstart еще раз, и посмотреть результат на окне Console, программа распечатает список файлов и папок на вашем Google Drive.
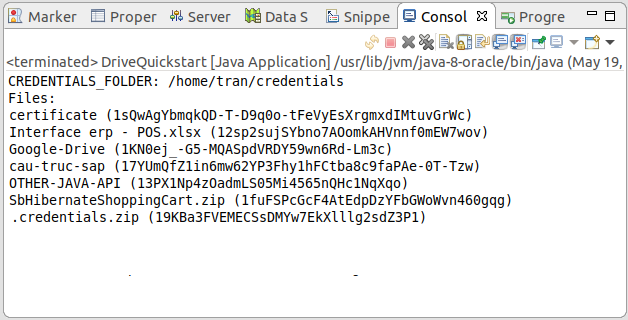
5. Структура File и Directory у Google Drive
Понятие про папку (Directory) и файл (File) вGoogle Drive немного отличается с понятием Directory & File на операционной системе. Ниже являются главные особенности:
- В Google Drive один File/Directory может иметь один или более родительский Directory.
- В одной и той же папке, файлы могут иметь одинаковые файлы, но разные ID.
- Класс com.google.api.services.drive.model.File является представителем обоих, File и Directory.
Класс com.google.api.services.drive.model.File имеет очень много полей (field) как в иллюстрационном изображении ниже:
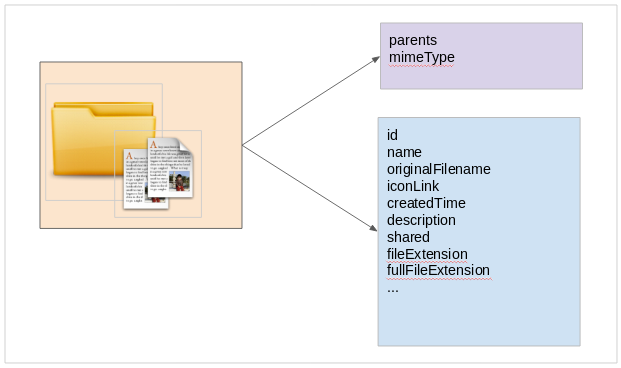
Имеется 2 важных поля (field) это mineType & parents:
parents
Список ID настоящих родительских папок файла (папки). Коренные (root) папки или файлы имеют родительскую папку с ID = "root".
mineType
application/vnd.google-apps.audio | |
application/vnd.google-apps.document | Google Docs |
application/vnd.google-apps.drawing | Google Drawing |
application/vnd.google-apps.file | Google Drive file |
application/vnd.google-apps.folder | Google Drive folder |
application/vnd.google-apps.form | Google Forms |
application/vnd.google-apps.fusiontable | Google Fusion Tables |
application/vnd.google-apps.map | Google My Maps |
application/vnd.google-apps.photo | |
application/vnd.google-apps.presentation | Google Slides |
application/vnd.google-apps.script | Google Apps Scripts |
application/vnd.google-apps.site | Google Sites |
application/vnd.google-apps.spreadsheet | Google Sheets |
application/vnd.google-apps.unknown | |
application/vnd.google-apps.video | |
application/vnd.google-apps.drive-sdk | 3rd party shortcut |
..... |
Операторы используемые для полей (field):
name | string | contains, =, != | Name of the file. |
fullText | string | contains | Full text of the file including name, description, content, and indexable text. |
mimeType | string | contains, =, != | MIME type of the file. |
modifiedTime | date | <=, <, =, !=, >, >= | Date of the last modification of the file. |
viewedByMeTime | date | <=, <, =, !=, >, >= | Date that the user last viewed a file. |
trashed | boolean | =, != | Whether the file is in the trash or not. |
starred | boolean | =, != | Whether the file is starred or not. |
parents | collection | in | Whether the parents collection contains the specified ID. |
owners | collection | in | Users who own the file. |
writers | collection | in | Users or groups who have permission to modify the file. |
readers | collection | in | Users or groups who have permission to read the file. |
sharedWithMe | boolean | =, != | Files that are in the user's "Shared with me" collection. |
properties | collection | has | Public custom file properties. |
appProperties | collection | has | Private custom file properties. |
visibility | string | =, '!=' | The visibility level of the file. Valid values are anyoneCanFind, anyoneWithLink, domainCanFind, domainWithLink, and limited. |
6. Класс GoogleDriveUtils
GoogleDriveUtils.java
package org.o7planning.googledrive.utils;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.Collections;
import java.util.List;
import com.google.api.client.auth.oauth2.Credential;
import com.google.api.client.extensions.java6.auth.oauth2.AuthorizationCodeInstalledApp;
import com.google.api.client.extensions.jetty.auth.oauth2.LocalServerReceiver;
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.http.HttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.jackson2.JacksonFactory;
import com.google.api.client.util.store.FileDataStoreFactory;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.DriveScopes;
public class GoogleDriveUtils {
private static final String APPLICATION_NAME = "Google Drive API Java Quickstart";
private static final JsonFactory JSON_FACTORY = JacksonFactory.getDefaultInstance();
// Directory to store user credentials for this application.
private static final java.io.File CREDENTIALS_FOLDER //
= new java.io.File(System.getProperty("user.home"), "credentials");
private static final String CLIENT_SECRET_FILE_NAME = "client_secret.json";
private static final List<String> SCOPES = Collections.singletonList(DriveScopes.DRIVE);
// Global instance of the {@link FileDataStoreFactory}.
private static FileDataStoreFactory DATA_STORE_FACTORY;
// Global instance of the HTTP transport.
private static HttpTransport HTTP_TRANSPORT;
private static Drive _driveService;
static {
try {
HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
DATA_STORE_FACTORY = new FileDataStoreFactory(CREDENTIALS_FOLDER);
} catch (Throwable t) {
t.printStackTrace();
System.exit(1);
}
}
public static Credential getCredentials() throws IOException {
java.io.File clientSecretFilePath = new java.io.File(CREDENTIALS_FOLDER, CLIENT_SECRET_FILE_NAME);
if (!clientSecretFilePath.exists()) {
throw new FileNotFoundException("Please copy " + CLIENT_SECRET_FILE_NAME //
+ " to folder: " + CREDENTIALS_FOLDER.getAbsolutePath());
}
InputStream in = new FileInputStream(clientSecretFilePath);
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in));
// Build flow and trigger user authorization request.
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY,
clientSecrets, SCOPES).setDataStoreFactory(DATA_STORE_FACTORY).setAccessType("offline").build();
Credential credential = new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user");
return credential;
}
public static Drive getDriveService() throws IOException {
if (_driveService != null) {
return _driveService;
}
Credential credential = getCredentials();
//
_driveService = new Drive.Builder(HTTP_TRANSPORT, JSON_FACTORY, credential) //
.setApplicationName(APPLICATION_NAME).build();
return _driveService;
}
}
7. SubFolder & RootFolder
Если вы знаете ID папки (Directory) вы можете получить список подпапок данной папки. Обратите внимание что в Google Drive файл (или папка) может иметь одну или много родительских папок.
Теперь, посмотрите пример получения списка подпапок одной папки, и пример получения списка корневых папок.
GetSubFolders.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
public class GetSubFolders {
// com.google.api.services.drive.model.File
public static final List<File> getGoogleSubFolders(String googleFolderIdParent) throws IOException {
Drive driveService = GoogleDriveUtils.getDriveService();
String pageToken = null;
List<File> list = new ArrayList<File>();
String query = null;
if (googleFolderIdParent == null) {
query = " mimeType = 'application/vnd.google-apps.folder' " //
+ " and 'root' in parents";
} else {
query = " mimeType = 'application/vnd.google-apps.folder' " //
+ " and '" + googleFolderIdParent + "' in parents";
}
do {
FileList result = driveService.files().list().setQ(query).setSpaces("drive") //
// Fields will be assigned values: id, name, createdTime
.setFields("nextPageToken, files(id, name, createdTime)")//
.setPageToken(pageToken).execute();
for (File file : result.getFiles()) {
list.add(file);
}
pageToken = result.getNextPageToken();
} while (pageToken != null);
//
return list;
}
// com.google.api.services.drive.model.File
public static final List<File> getGoogleRootFolders() throws IOException {
return getGoogleSubFolders(null);
}
public static void main(String[] args) throws IOException {
List<File> googleRootFolders = getGoogleRootFolders();
for (File folder : googleRootFolders) {
System.out.println("Folder ID: " + folder.getId() + " --- Name: " + folder.getName());
}
}
}
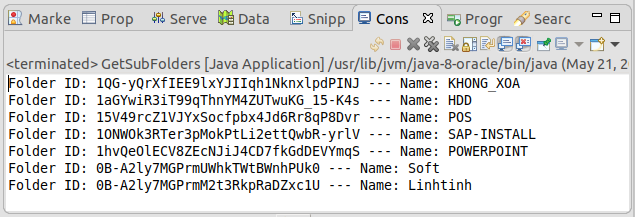
Примечание: У вас есть объект com.google.api.services.drive.model.File, но не все его поля (field) имеют прикрепленное значение. Только поля, в которых вы заинтересованы имеют прикрепленные значение, если наоборот то имеет значение null.FileList result = driveService.files().list().setQ(query).setSpaces("drive") // // Fields will be assigned values: id, name, createdTime .setFields("nextPageToken, files(id, name, createdTime)")// .setPageToken(pageToken).execute();
Следующий пример, поиск папок по имени , которые являются подпапками определенной папки.
GetSubFoldersByName.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
public class GetSubFoldersByName {
// com.google.api.services.drive.model.File
public static final List<File> getGoogleSubFolderByName(String googleFolderIdParent, String subFolderName)
throws IOException {
Drive driveService = GoogleDriveUtils.getDriveService();
String pageToken = null;
List<File> list = new ArrayList<File>();
String query = null;
if (googleFolderIdParent == null) {
query = " name = '" + subFolderName + "' " //
+ " and mimeType = 'application/vnd.google-apps.folder' " //
+ " and 'root' in parents";
} else {
query = " name = '" + subFolderName + "' " //
+ " and mimeType = 'application/vnd.google-apps.folder' " //
+ " and '" + googleFolderIdParent + "' in parents";
}
do {
FileList result = driveService.files().list().setQ(query).setSpaces("drive") //
.setFields("nextPageToken, files(id, name, createdTime)")//
.setPageToken(pageToken).execute();
for (File file : result.getFiles()) {
list.add(file);
}
pageToken = result.getNextPageToken();
} while (pageToken != null);
//
return list;
}
// com.google.api.services.drive.model.File
public static final List<File> getGoogleRootFoldersByName(String subFolderName) throws IOException {
return getGoogleSubFolderByName(null,subFolderName);
}
public static void main(String[] args) throws IOException {
List<File> rootGoogleFolders = getGoogleRootFoldersByName("TEST");
for (File folder : rootGoogleFolders) {
System.out.println("Folder ID: " + folder.getId() + " --- Name: " + folder.getName());
}
}
}
8. Search Files
Чтобы найти файлы на Google Drive, вам следует использовать следующее условие запроса:
- mimeType != 'application/vnd.google-apps.folder'
FindFilesByName.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
public class FindFilesByName {
// com.google.api.services.drive.model.File
public static final List<File> getGoogleFilesByName(String fileNameLike) throws IOException {
Drive driveService = GoogleDriveUtils.getDriveService();
String pageToken = null;
List<File> list = new ArrayList<File>();
String query = " name contains '" + fileNameLike + "' " //
+ " and mimeType != 'application/vnd.google-apps.folder' ";
do {
FileList result = driveService.files().list().setQ(query).setSpaces("drive") //
// Fields will be assigned values: id, name, createdTime, mimeType
.setFields("nextPageToken, files(id, name, createdTime, mimeType)")//
.setPageToken(pageToken).execute();
for (File file : result.getFiles()) {
list.add(file);
}
pageToken = result.getNextPageToken();
} while (pageToken != null);
//
return list;
}
public static void main(String[] args) throws IOException {
List<File> rootGoogleFolders = getGoogleFilesByName("u");
for (File folder : rootGoogleFolders) {
System.out.println("Mime Type: " + folder.getMimeType() + " --- Name: " + folder.getName());
}
System.out.println("Done!");
}
}
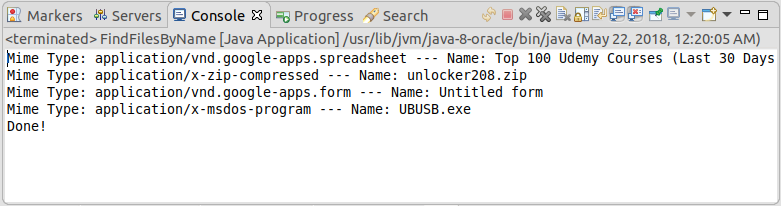
9. Создать папку на Google Drive
CreateFolder.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
public class CreateFolder {
public static final File createGoogleFolder(String folderIdParent, String folderName) throws IOException {
File fileMetadata = new File();
fileMetadata.setName(folderName);
fileMetadata.setMimeType("application/vnd.google-apps.folder");
if (folderIdParent != null) {
List<String> parents = Arrays.asList(folderIdParent);
fileMetadata.setParents(parents);
}
Drive driveService = GoogleDriveUtils.getDriveService();
// Create a Folder.
// Returns File object with id & name fields will be assigned values
File file = driveService.files().create(fileMetadata).setFields("id, name").execute();
return file;
}
public static void main(String[] args) throws IOException {
// Create a Root Folder
File folder = createGoogleFolder(null, "TEST-FOLDER");
System.out.println("Created folder with id= "+ folder.getId());
System.out.println(" name= "+ folder.getName());
System.out.println("Done!");
}
}
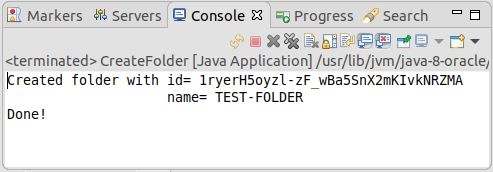
10. Создать файл на Google Drive
Есть 3 распространенных способа для того, чтобы вы создали файл на Google Driver:
- Upload File
- byte[]
- InputStream
CreateGoogleFile.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.io.InputStream;
import java.util.Arrays;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.client.http.AbstractInputStreamContent;
import com.google.api.client.http.ByteArrayContent;
import com.google.api.client.http.FileContent;
import com.google.api.client.http.InputStreamContent;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
public class CreateGoogleFile {
// PRIVATE!
private static File _createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, AbstractInputStreamContent uploadStreamContent) throws IOException {
File fileMetadata = new File();
fileMetadata.setName(customFileName);
List<String> parents = Arrays.asList(googleFolderIdParent);
fileMetadata.setParents(parents);
//
Drive driveService = GoogleDriveUtils.getDriveService();
File file = driveService.files().create(fileMetadata, uploadStreamContent)
.setFields("id, webContentLink, webViewLink, parents").execute();
return file;
}
// Create Google File from byte[]
public static File createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, byte[] uploadData) throws IOException {
//
AbstractInputStreamContent uploadStreamContent = new ByteArrayContent(contentType, uploadData);
//
return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent);
}
// Create Google File from java.io.File
public static File createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, java.io.File uploadFile) throws IOException {
//
AbstractInputStreamContent uploadStreamContent = new FileContent(contentType, uploadFile);
//
return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent);
}
// Create Google File from InputStream
public static File createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, InputStream inputStream) throws IOException {
//
AbstractInputStreamContent uploadStreamContent = new InputStreamContent(contentType, inputStream);
//
return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent);
}
public static void main(String[] args) throws IOException {
java.io.File uploadFile = new java.io.File("/home/tran/Downloads/test.txt");
// Create Google File:
File googleFile = createGoogleFile(null, "text/plain", "newfile.txt", uploadFile);
System.out.println("Created Google file!");
System.out.println("WebContentLink: " + googleFile.getWebContentLink() );
System.out.println("WebViewLink: " + googleFile.getWebViewLink() );
System.out.println("Done!");
}
}
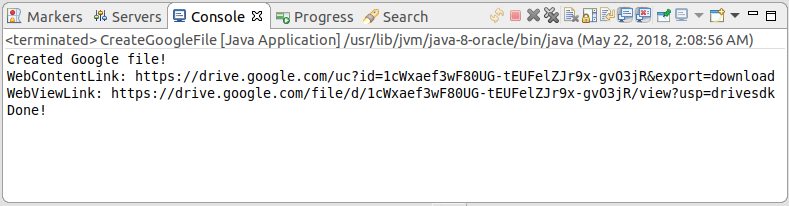
11. Поделиться Google File/Folder
ShareGoogleFile.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.Permission;
public class ShareGoogleFile {
// Public a Google File/Folder.
public static Permission createPublicPermission(String googleFileId) throws IOException {
// All values: user - group - domain - anyone
String permissionType = "anyone";
// All values: organizer - owner - writer - commenter - reader
String permissionRole = "reader";
Permission newPermission = new Permission();
newPermission.setType(permissionType);
newPermission.setRole(permissionRole);
Drive driveService = GoogleDriveUtils.getDriveService();
return driveService.permissions().create(googleFileId, newPermission).execute();
}
public static Permission createPermissionForEmail(String googleFileId, String googleEmail) throws IOException {
// All values: user - group - domain - anyone
String permissionType = "user"; // Valid: user, group
// organizer - owner - writer - commenter - reader
String permissionRole = "reader";
Permission newPermission = new Permission();
newPermission.setType(permissionType);
newPermission.setRole(permissionRole);
newPermission.setEmailAddress(googleEmail);
Drive driveService = GoogleDriveUtils.getDriveService();
return driveService.permissions().create(googleFileId, newPermission).execute();
}
public static void main(String[] args) throws IOException {
String googleFileId1 = "some-google-file-id-1";
String googleEmail = "test.o7planning@gmail.com";
// Share for a User
createPermissionForEmail(googleFileId1, googleEmail);
String googleFileId2 = "some-google-file-id-2";
// Share for everyone
createPublicPermission(googleFileId2);
System.out.println("Done!");
}
}
Java Open source libraries
- Руководство Java JSON Processing API (JSONP)
- Использование Scribe OAuth Java API с Google OAuth 2
- Получить информацию об оборудовании в приложении Java
- Restfb Java API для Facebook
- Создание Credentials для Google Drive API
- Руководство Java JDOM2
- Руководство Java XStream
- Использование Java JSoup для анализа кода HTML
- Получение географической информации на основе IP-адреса с помощью GeoIP2 Java API
- Чтение и запись файла Excel в Java с использованием Apache POI
- Изучите Facebook Graph API
- Манипулирование файлами и папками в Google Drive с использованием Java
Show More