Руководство Java SWT TabFolder и CTabFolder
1. TabFolder и CTabFolder
В SWT,TabFolder является подклассом Composite. Каждый TabFolder может содержать много TabItem В то же время пользователь может видеть только один TabItem.
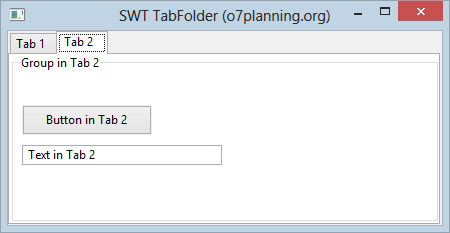
CTabFolder это расширенный класс от класса Composite, похож на TabFolder.CTabFolder содержит один или много CTabItem, и вы можете закрыть CTabItem.
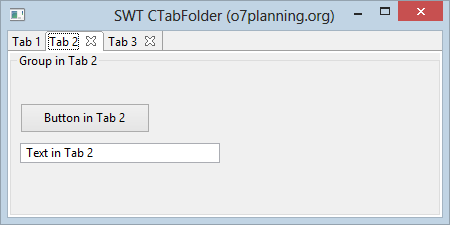
2. Например TabFolder
Следуюший пример, TabFolder с 2 Tab
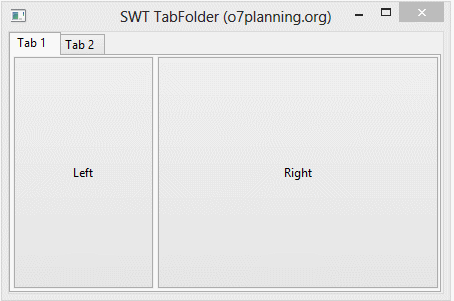
TabFolderDemo.java
package org.o7planning.swt.tabfolder;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.SashForm;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.TabFolder;
import org.eclipse.swt.widgets.TabItem;
import org.eclipse.swt.widgets.Text;
public class TabFolderDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT TabFolder (o7planning.org)");
shell.setSize(450, 300);
shell.setLayout(new FillLayout());
TabFolder folder = new TabFolder(shell, SWT.NONE);
// Tab 1
TabItem tab1 = new TabItem(folder, SWT.NONE);
tab1.setText("Tab 1");
// Create the HORIZONTAL SashForm.
SashForm sashForm = new SashForm(folder, SWT.HORIZONTAL);
new Button(sashForm, SWT.PUSH).setText("Left");
new Button(sashForm, SWT.PUSH).setText("Right");
sashForm.setWeights(new int[]{1,2});
tab1.setControl(sashForm);
// Tab 2
TabItem tab2 = new TabItem(folder, SWT.NONE);
tab2.setText("Tab 2");
Group group = new Group(folder, SWT.NONE);
group.setText("Group in Tab 2");
Button button = new Button(group, SWT.NONE);
button.setText("Button in Tab 2");
button.setBounds(10, 50, 130, 30);
Text text = new Text(group, SWT.BORDER);
text.setText("Text in Tab 2");
text.setBounds(10, 90, 200, 20);
tab2.setControl(group);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
3. Пример CTabFolder
CTabFolder это кастомизированнай tab folder. CTabItem может применить стиль SWT.CLOSE, позволяет пользователям закрыть этот CTabItem.
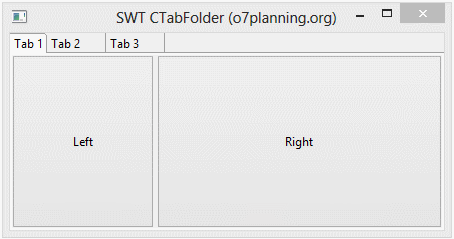
CTabFolderDemo.java
package org.o7planning.swt.tabfolder;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.CTabFolder;
import org.eclipse.swt.custom.CTabItem;
import org.eclipse.swt.custom.SashForm;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class CTabFolderDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT CTabFolder (o7planning.org)");
shell.setSize(450, 300);
shell.setLayout(new FillLayout());
CTabFolder folder = new CTabFolder(shell, SWT.NONE);
// Tab 1
CTabItem tab1 = new CTabItem(folder, SWT.NONE);
tab1.setText("Tab 1");
// Create the HORIZONTAL SashForm.
SashForm sashForm = new SashForm(folder, SWT.HORIZONTAL);
new Button(sashForm, SWT.PUSH).setText("Left");
new Button(sashForm, SWT.PUSH).setText("Right");
sashForm.setWeights(new int[] { 1, 2 });
tab1.setControl(sashForm);
// Tab 2 with close icon
CTabItem tab2 = new CTabItem(folder, SWT.NONE | SWT.CLOSE);
tab2.setText("Tab 2");
Group group = new Group(folder, SWT.NONE);
group.setText("Group in Tab 2");
Button button = new Button(group, SWT.NONE);
button.setText("Button in Tab 2");
button.setBounds(10, 50, 130, 30);
Text text = new Text(group, SWT.BORDER);
text.setText("Text in Tab 2");
text.setBounds(10, 90, 200, 20);
tab2.setControl(group);
// Tab 3 with close icon
CTabItem tab3 = new CTabItem(folder, SWT.NONE | SWT.CLOSE);
tab3.setText("Tab 3");
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
4. TabFolder & Event
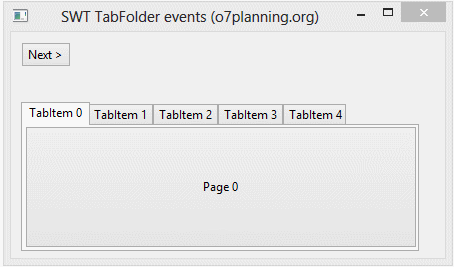
TabFolderEventsExample.java
package org.o7planning.swt.tabfolder;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.TabFolder;
import org.eclipse.swt.widgets.TabItem;
public class TabFolderEventsExample {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT TabFolder events (o7planning.org)");
shell.setSize(450, 300);
shell.setLayout(null);
Button buttonNext = new Button(shell, SWT.NONE);
buttonNext.setText("Next >");
buttonNext.setBounds(10, 10, 50, 25);
// Log
Label labelLog = new Label(shell, SWT.NONE);
labelLog.setBounds(10, 40, 400, 20);
// TabFolder
TabFolder tabFolder = new TabFolder(shell, SWT.NONE);
tabFolder.setBounds(10, 70, 400, 150);
for (int i = 0; i < 5; i++) {
TabItem item = new TabItem(tabFolder, SWT.NONE);
item.setText("TabItem " + i);
Button button = new Button(tabFolder, SWT.PUSH);
button.setText("Page " + i);
item.setControl(button);
}
// Events
tabFolder.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent evt) {
TabItem item = tabFolder.getSelection()[0];
labelLog.setText(item.getText() + " selected!");
}
});
buttonNext.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent evt) {
int idx = tabFolder.getSelectionIndex();
if (idx + 1 == tabFolder.getItemCount()) {
idx = -1;
}
tabFolder.setSelection(idx + 1);
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
5. CTabFolder & Event
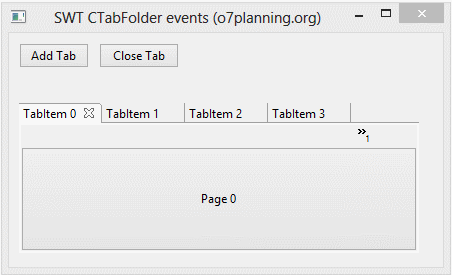
CTabFolderEventsExample.java
package org.o7planning.swt.tabfolder;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.CTabFolder;
import org.eclipse.swt.custom.CTabItem;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class CTabFolderEventsExample {
private static int nextIndex = 6;
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT CTabFolder events (o7planning.org)");
shell.setSize(450, 300);
shell.setLayout(null);
Button buttonAdd = new Button(shell, SWT.NONE);
buttonAdd.setText("Add Tab");
buttonAdd.setBounds(10, 10, 70, 25);
Button buttonClose = new Button(shell, SWT.NONE);
buttonClose.setText("Close Tab");
buttonClose.setBounds(90, 10, 80, 25);
// CTabFolder
CTabFolder ctabFolder = new CTabFolder(shell, SWT.NONE);
ctabFolder.setBounds(10, 70, 400, 150);
for (int i = 0; i < 5; i++) {
CTabItem item = new CTabItem(ctabFolder, SWT.CLOSE);
item.setText("TabItem " + i);
Button button = new Button(ctabFolder, SWT.PUSH);
button.setText("Page " + i);
item.setControl(button);
}
ctabFolder.setSelection(0);
// Add Tab
buttonAdd.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent evt) {
int idx = ctabFolder.getSelectionIndex();
CTabItem item = new CTabItem(ctabFolder, SWT.CLOSE, idx + 1);
item.setText("TabItem " + nextIndex++);
}
});
// Close Tab
buttonClose.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent evt) {
CTabItem item = ctabFolder.getSelection();
if (item != null) {
item.dispose();
}
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Руководства Java SWT
- Руководство Java SWT FillLayout
- Руководство Java SWT RowLayout
- Руководство Java SWT SashForm
- Руководство Java SWT Label
- Руководство Java SWT Button
- Руководство Java SWT Toggle Button
- Руководство Java SWT Radio Button
- Руководство Java SWT Text
- Руководство Java SWT Password Field
- Руководство Java SWT Link
- Программирование приложения Java Desktop с использованием SWT
- Руководство Java SWT Combo
- Руководство Java SWT Spinner
- Руководство Java SWT Slider
- Руководство Java SWT Scale
- Руководство Java SWT ProgressBar
- Руководство Java SWT TabFolder и CTabFolder
- Руководство Java SWT List
Show More