Руководство Java String, StringBuffer и StringBuilder
1. Иерархическое наследование
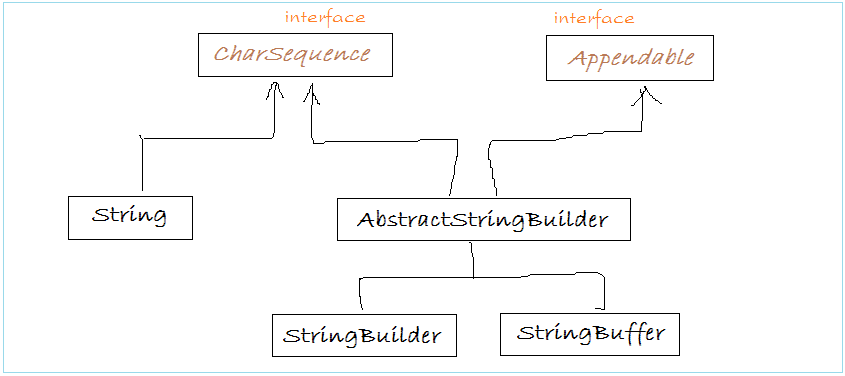
При работе с текстовым данными, Java предоставляет вам 3 класса String, StringBuffer и StringBuilder. При работе с большими данными вам стоит использовать StringBuffer или StringBuilder чтобы достичь лучшую эффективность. Стандартно эти 3 класса имеют много общего.
StringBuilder иStringBuffer похожи, лишь отличаются в ситуациях связанные с использованием многопоточности (Multi Thread).
- String не изменяется (immutable), это понятие будет детально описано в статье. Он не позволяет иметь подкласс.
- StringBuffer, StringBuilder могут быть изменены (mutable)
StringBuilder иStringBuffer похожи, лишь отличаются в ситуациях связанные с использованием многопоточности (Multi Thread).
- При обработке тектса со многими потоками(Thread) вам следует использовать StringBuffer, чтобы предотвратить конфликт между потоками.
- При обработке текста с одним потоком стоит использовать StringBuilder.
2. Понятия изменяемые & неизменяемые
Посмотрим иллюстрированный пример:
// This is a class with value field and name field.
// When you create this class, you cannot reset the value and all other fields from outside.
// This class does not have methods for the purpose of resetting fields from outside.
// It means this class is immutable
public class ImmutableClassExample {
private int value;
private String name;
public ImmutableClassExample(String name, int value) {
this.value = value;
this.name= name;
}
public String getName() {
return name;
}
public int getValue() {
return value;
}
}
// This is a class owning a value field.
// After creating the object, you can reset values of the value field by calling setNewValue(int) method.
// This is a mutable class.
public class MutableClassExample {
private int value;
public MutableClassExample(int value) {
this.value= value;
}
public void setNewValue(int newValue) {
this.value = newValue;
}
}
String это неизменяемый класс, String имеет разные свойства (поля), например length,... го эти значения не могут быть изменены.
3. String
String это очень важный класс в Java, и любой кто начинает с Java использует команду System.out.println() чтобы распечатать String на экран Console. Многие обычно не знают что String является неизменным (immutable) и финальным (Не позволяет классу наследовать его), все изменения в String создают другой объект String.
String is a very special class
В java, String это особенный класс, причиной является то, что он используется постоянно в программе, поэтому он трребует производительность и гибкость. Это причина, почему String имеет свойства объекта и примитивный вид (primitive).
Примитивный вид:
Вы можете создать string literal (строковый литерал), string literal хранится в стеке (stack), требует пространство хранения, и дешевле при обработке.
String literal хранятся в общем пуле (common pool). Так два string literal имеют похожее содержание, используют общее место в памяти в стеке, это помогает сэкономить память.
- String literal = "Hello World";
String literal хранятся в общем пуле (common pool). Так два string literal имеют похожее содержание, используют общее место в памяти в стеке, это помогает сэкономить память.
Свойство объекта:
Так как String является классом, он может быть создан используя оператор new.
- String object = new String("Hello World");
Например:
// Implicit construction via string literal
String str1 = "Java is Hot";
// Explicit construction via new
String str2 = new String("I'm cool");
String Literal vs. String Object
Как упомянуто, существует два способа построить строку (String): неявное строительство путем назначения строкового литерала (String literal) или явно создать объект String через оператор new и составляющие String. Например,
String s1 = "Hello"; // String literal
String s2 = "Hello"; // String literal
String s3 = s1; // same reference
String s4 = new String("Hello"); // String object
String s5 = new String("Hello"); // String object
Мы объясним, исопльзуя иллюстрации ниже:
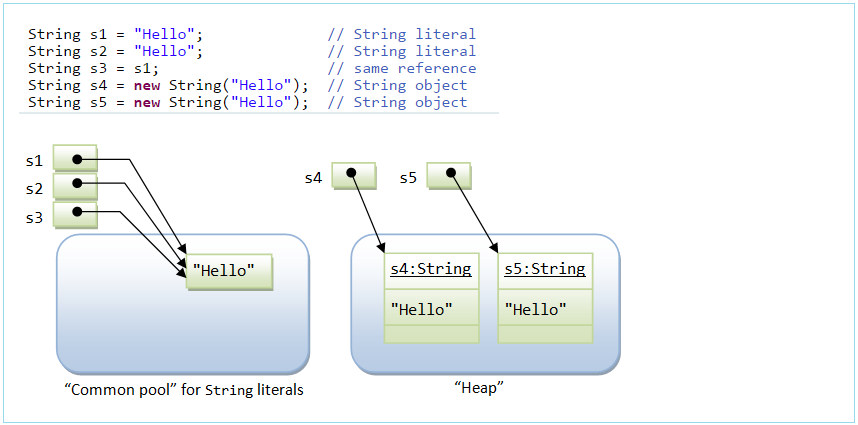
String literal с одинаковым содержанием, они разделят одно место хранения в общем пуле (common pool). При этом объекты String хранятся в Heap, и не разделяют место хранения включая эти 2 объекта string с одинаковым содержанием.
equals() vs ==
Метод equals() используется для сравнения 2-х объектов, с String это означает сравнение содержания 2-х string. Для ссылочных видов (reference), оператор == значит сравнение адреса области хранения объекта. Посмотрим пример:
String s1 = "Hello"; // String literal
String s2 = "Hello"; // String literal
String s3 = s1; // same reference
String s4 = new String("Hello"); // String object
String s5 = new String("Hello"); // String object
s1 == s1; // true, same pointer
s1 == s2; // true, s1 and s2 share storage in common pool
s1 == s3; // true, s3 is assigned same pointer as s1
s1 == s4; // false, different pointers
s4 == s5; // false, different pointers in heap
s1.equals(s3); // true, same contents
s1.equals(s4); // true, same contents
s4.equals(s5); // true, same contents
На самом деле, вам следует использовать String literal, вместо оператора new. Это ускоряет вашу программу.
The methods of the String
Далее идет список методов String.
SN | Methods | Description |
1 | char charAt(int index) | Returns the character at the specified index. |
2 | int compareTo(Object o) | Compares this String to another Object. |
3 | int compareTo(String anotherString) | Compares two strings lexicographically. |
4 | int compareToIgnoreCase(String str) | Compares two strings lexicographically, ignoring case differences. |
5 | String concat(String str) | Concatenates the specified string to the end of this string. |
6 | boolean contentEquals(StringBuffer sb) | Returns true if and only if this String represents the same sequence of characters as the specified StringBuffer. |
7 | static String copyValueOf(char[] data) | Returns a String that represents the character sequence in the array specified. |
8 | static String copyValueOf(char[] data, int offset, int count) | Returns a String that represents the character sequence in the array specified. |
9 | boolean endsWith(String suffix) | Tests if this string ends with the specified suffix. |
10 | boolean equals(Object anObject) | Compares this string to the specified object. |
11 | boolean equalsIgnoreCase(String anotherString) | Compares this String to another String, ignoring case considerations. |
12 | byte getBytes() | Encodes this String into a sequence of bytes using the platform's default charset, storing the result into a new byte array. |
13 | byte[] getBytes(String charsetName) | Encodes this String into a sequence of bytes using the named charset, storing the result into a new byte array. |
14 | void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin) | Copies characters from this string into the destination character array. |
15 | int hashCode() | Returns a hash code for this string. |
16 | int indexOf(int ch) | Returns the index within this string of the first occurrence of the specified character. |
17 | int indexOf(int ch, int fromIndex) | Returns the index within this string of the first occurrence of the specified character, starting the search at the specified index. |
18 | int indexOf(String str) | Returns the index within this string of the first occurrence of the specified substring. |
19 | int indexOf(String str, int fromIndex) | Returns the index within this string of the first occurrence of the specified substring, starting at the specified index. |
20 | String intern() | Returns a canonical representation for the string object. |
21 | int lastIndexOf(int ch) | Returns the index within this string of the last occurrence of the specified character. |
22 | int lastIndexOf(int ch, int fromIndex) | Returns the index within this string of the last occurrence of the specified character, searching backward starting at the specified index. |
23 | int lastIndexOf(String str) | Returns the index within this string of the rightmost occurrence of the specified substring. |
24 | int lastIndexOf(String str, int fromIndex) | Returns the index within this string of the last occurrence of the specified substring, searching backward starting at the specified index. |
25 | int length() | Returns the length of this string. |
26 | boolean matches(String regex) | Tells whether or not this string matches the given regular expression. |
27 | boolean regionMatches(boolean ignoreCase, int toffset, String other, int ooffset, int len) | Tests if two string regions are equal. |
28 | boolean regionMatches(int toffset, String other, int ooffset, int len) | Tests if two string regions are equal. |
29 | String replace(char oldChar, char newChar) | Returns a new string resulting from replacing all occurrences of oldChar in this string with newChar. |
30 | String replaceAll(String regex, String replacement) | Replaces each substring of this string that matches the given regular expression with the given replacement. |
31 | String replaceFirst(String regex, String replacement) | Replaces the first substring of this string that matches the given regular expression with the given replacement. |
32 | String[] split(String regex) | Splits this string around matches of the given regular expression. |
33 | String[] split(String regex, int limit) | Splits this string around matches of the given regular expression. |
34 | boolean startsWith(String prefix) | Tests if this string starts with the specified prefix. |
35 | boolean startsWith(String prefix, int toffset) | Tests if this string starts with the specified prefix beginning a specified index. |
36 | CharSequence subSequence(int beginIndex, int endIndex) | Returns a new character sequence that is a subsequence of this sequence. |
37 | String substring(int beginIndex) | Returns a new string that is a substring of this string. |
38 | String substring(int beginIndex, int endIndex) | Returns a new string that is a substring of this string. |
39 | char[] toCharArray() | Converts this string to a new character array. |
40 | String toLowerCase() | Converts all of the characters in this String to lower case using the rules of the default locale. |
41 | String toLowerCase(Locale locale) | Converts all of the characters in this String to lower case using the rules of the given Locale. |
42 | String toString() | This object (which is already a string!) is itself returned. |
43 | String toUpperCase() | Converts all of the characters in this String to upper case using the rules of the default locale. |
44 | String toUpperCase(Locale locale) | Converts all of the characters in this String to upper case using the rules of the given Locale. |
45 | String trim() | Returns a copy of the string, with leading and trailing whitespace omitted. |
46 | static String valueOf(primitive data type x) | Returns the string representation of the passed data type argument. |
4. StringBuffer vs StringBuilder
StringBuilder и StringBuffer очень похожи, отличие в том, что все методы StringBuffer синхронизированы, он подходит, когда вы работаете с многопоточным приложением, несколько потоков могут одновременно иметь доступ в объект StringBuffer. В это време, StringBuilder имеет похожие методы но не синхронизированы, но его производительность выше. Вам следует использовать StringBuilder в однопоточном приложении, или использовать как локальную переменную в методе.
Методы StringBuffer (StringBuilder индентичны)
// Constructors
StringBuffer() // an initially-empty StringBuffer
StringBuffer(int size) // with the specified initial size
StringBuffer(String s) // with the specified initial content
// Length
int length()
// Methods for building up the content
// type could be primitives, char[], String, StringBuffer, etc
StringBuffer append(type arg) // ==> note above!
StringBuffer insert(int offset, type arg) // ==> note above!
// Methods for manipulating the content
StringBuffer delete(int start, int end)
StringBuffer deleteCharAt(int index)
void setLength(int newSize)
void setCharAt(int index, char newChar)
StringBuffer replace(int start, int end, String s)
StringBuffer reverse()
// Methods for extracting whole/part of the content
char charAt(int index)
String substring(int start)
String substring(int start, int end)
String toString()
// Methods for searching
int indexOf(String searchKey)
int indexOf(String searchKey, int fromIndex)
int lastIndexOf(String searchKey)
int lastIndexOf(String searchKey, int fromIndex)
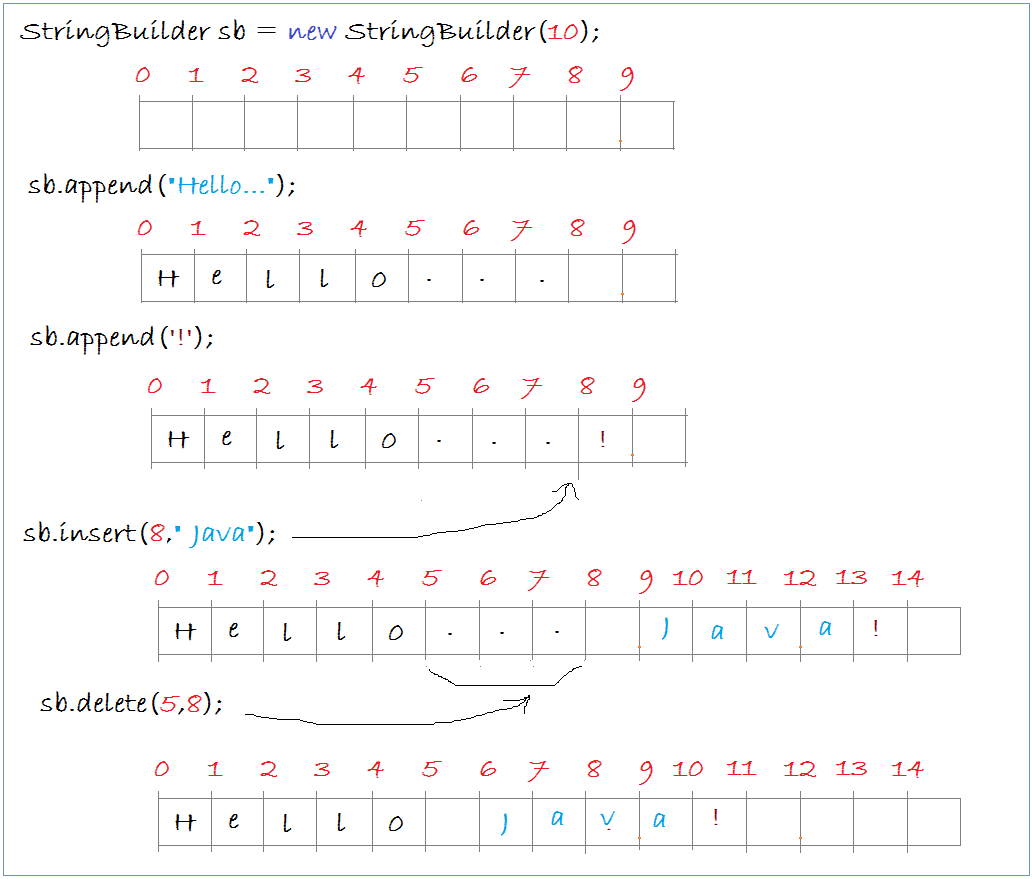
StringBuilderDemo.java
package org.o7planning.tutorial.strbb;
public class StringBuilderDemo {
public static void main(String[] args) {
// Create StringBuilder object
// with no characters in it and
// an initial capacity specified by the capacity argument
StringBuilder sb = new StringBuilder(10);
// Append the string Hello ... on sb.
sb.append("Hello...");
System.out.println("- sb after appends a string: " + sb);
// append a character
char c = '!';
sb.append(c);
System.out.println("- sb after appending a char: " + sb);
// Insert a string at index 5
sb.insert(8, " Java");
System.out.println("- sb after insert string: " + sb);
// Delete substring at index 5 to 8
sb.delete(5,8);
System.out.println("- sb after delete: " + sb);
}
}
Результаты запуска примера:
- sb after appends a string: Hello...
- sb after appending a char: Hello...!
- sb after insert string: Hello... Java!
- sb after delete: Hello Java!
Java Basic
- Настройте java compiler для обработки вашего Annotation (Annotation Processing Tool)
- Программирование на Java для группы с помощью Eclipse и SVN
- Руководство Java WeakReference
- Руководство Java PhantomReference
- Сжатие и декомпрессия в Java
- Настройка Eclipse для использования JDK вместо JRE
- Методы String.format() и printf() в Java
- Синтаксис и новые функции в Java 8
- Регулярные выражения Java
- Руководство Java Multithreading Programming
- Библиотеки Java JDBC Driver для различных типов баз данных
- Руководство Java JDBC
- Получить значения столбцов, автоматически возрастающих при вставлении (Insert) записи, используя JDBC
- Руководство Java Stream
- Руководство Java Functional Interface
- Введение в Raspberry Pi
- Руководство Java Predicate
- Абстрактный класс и Interface в Java
- Модификатор доступа (Access modifiers) в Java
- Руководство Java Enum
- Руководство Java Annotation
- Сравнение и Сортировка в Java
- Руководство Java String, StringBuffer и StringBuilder
- Обработка исключений Java - Java Exception Handling
- Руководство Java Generics
- Манипулирование файлами и каталогами в Java
- Руководство Java BiPredicate
- Руководство Java Consumer
- Руководство Java BiConsumer
- Что мне нужно для начала работы с Java?
- История Java и разница между Oracle JDK и OpenJDK
- Установить Java в Windows
- Установите Java в Ubuntu
- Установите OpenJDK в Ubuntu
- Установить Eclipse
- Установите Eclipse в Ubuntu
- Быстрое изучение Java для начинающих
- История бит и байтов в информатике
- Типы данных в java
- Битовые операции
- Команда if else в Java
- команды switch в Java
- Циклы в Java
- Массивы (Array) в Java
- JDK Javadoc в формате CHM
- Наследование и полиморфизм в Java
- Руководство Java Function
- Руководство Java BiFunction
- Пример Java encoding и decoding с использованием Apache Base64
- Руководство Java Reflection
- Java Удаленный вызов методов - Java RMI
- Руководство Программирование Java Socket
- Какую платформу я должен выбрать для разработки приложений Java Desktop?
- Руководство Java Commons IO
- Руководство Java Commons Email
- Руководство Java Commons Logging
- Понимание Java System.identityHashCode, Object.hashCode и Object.equals
- Руководство Java SoftReference
- Руководство Java Supplier
- Аспектно-ориентированное программирование Java с помощью AspectJ (AOP)
Show More
- Руководства Java Servlet/JSP
- Руководства Java Collections Framework
- Java API для HTML, XML
- Руководства Java IO
- Руководства Java Date Time
- Руководства Spring Boot
- Руководства Maven
- Руководства Gradle
- Руководства Java Web Services
- Руководства Java SWT
- Руководства JavaFX
- Руководства Oracle Java ADF
- Руководства Struts2 Framework
- Руководства Spring Cloud