Методы String.format() и printf() в Java
1. printf()
В программировании на Java вы часто сталкиваетесь с кодом System.out.printf(..). На самом деле метод printf(..) определен как в классе PrintStream, так и в классе PrintWriter, их использование аналогично, printf означает "Print + Format".
printf methods
// Definition of the printf methods in the PrintWriter class:
public PrintStream printf(Locale l, String format, Object... args)
public PrintStream printf(String format, Object... args)
// Definition of the printf methods in PrintWriter class:
public PrintWriter printf(Locale l, String format, Object... args)
public PrintWriter format(String format, Object... args)
System.out.printf(..) - это особый случай, используемый для записи форматированного текста на экран Console, где System.out по сути является объектом типа PrintStream.
System class
package java.lang;
public final class System {
public static final PrintStream out = ...;
}
Прежде чем мы начнем, давайте рассмотрим простой пример:
Printf_ex1.java
package org.o7planning.printf.ex;
public class Printf_ex1 {
public static void main(String[] args) {
String firstName = "James";
String lastName = "Smith";
int age = 20;
System.out.printf("My name is %s %s, I'm %d years old.", firstName, lastName, age);
}
}
Output:
My name is James Smith, I'm 20 years old.
2. Правила форматирования
%[flags][width][.precision]conversion-character
Параметры: flags, width, precision являются опциональными.
3. conversion-character
%[flags][width][.precision]conversion-character
conversion-character | Description | Type |
d | decimal integer | byte, short, int, long |
f | floating-point number | float, double |
b | Boolean | Object |
B | will uppercase the boolean | Object |
c | Character Capital | String |
C | will uppercase the letter | String |
s | String Capital | String |
S | will uppercase all the letters in the string | String |
h | hashcode - A hashcode is like an address. This is useful for printing a reference | Object |
n | newline - Platform specific newline character - use %n instead of \n for greater compatibility |
conversion-character: n
System.out.printf("One%nTwo%nThree");
Output:
One
Two
Three
conversion-character: s
System.out.printf("My name is %s %s", "James", "Smith");
Output:
My name is James Smith
conversion-character: S
System.out.printf("My name is %S %S", "James", "Smith");
Output:
My name is JAMES SMITH
conversion-character: b
System.out.printf("%b%n", null);
System.out.printf("%b%n", false);
System.out.printf("%b%n", 5.3);
System.out.printf("%b%n", "Any text");
System.out.printf("%b%n", new Object());
Output:
false
false
true
true
true
conversion-character: B
System.out.printf("%B%n", null);
System.out.printf("%B%n", false);
System.out.printf("%B%n", 5.3);
System.out.printf("%B%n", "Any text");
System.out.printf("%B%n", new Object());
Output:
FALSE
FALSE
TRUE
TRUE
TRUE
conversion-character: d
System.out.printf("There are %d teachers and %d students in the class", 2, 25);
Output:
There are 2 teachers and 25 students in the class
conversion-character: f
System.out.printf("Exchange rate today: EUR %f = USD %f", 1.0, 1.2059);
Output:
Exchange rate today: EUR 1.000000 = USD 1.205900
conversion-character: c
char ch = 'a';
System.out.printf("Code of '%c' character is %d", ch, (int)ch);
Output:
Code of 'a' character is 97
conversion-character: C
char ch = 'a';
System.out.printf("'%C' is the upper case of '%c'", ch, ch);
Output:
'A' is the upper case of 'a'
conversion-character: h
Printf_cc_h_ex.java
package org.o7planning.printf.ex;
public class Printf_cc_h_ex {
public static void main(String[] args) {
// h (Hashcode HEX)
Object myObject = new AnyObject("Something");
System.out.println("Hashcode: " + myObject.hashCode());
System.out.println("Identity Hashcode: " + System.identityHashCode(myObject));
System.out.println("Hashcode (HEX): " + Integer.toHexString(myObject.hashCode()));
System.out.println("toString : " + String.valueOf(myObject));
System.out.printf("Printf: %h", myObject);
}
}
class AnyObject {
private String val;
public AnyObject(String val) {
this.val = val;
}
@Override
public int hashCode() {
if (this.val == null || this.val.isEmpty()) {
return 0;
}
return 1000 + (int) this.val.charAt(0);
}
}
Output:
Hashcode: 1083
Identity Hashcode: 1579572132
Hashcode (HEX): 43b
toString : org.o7planning.printf.ex.AnyObject@43b
Printf: 43b
4. width
%[flags][width][.precision]conversion-character
width - задает минимальное пространство (количество символов), которое требуется для печати содержимого соответствующего аргумента.
printf(format, arg1, arg2,.., argN)
5. flags
%[flags][width][.precision]conversion-character
flag | Description |
- | left-justify (default is to right-justify ) |
+ | output a plus ( + ) or minus ( - ) sign for a numerical value |
0 | forces numerical values to be zero-padded (default is blank padding ) |
, | comma grouping separator (for numbers > 1000) |
space will display a minus sign if the number is negative or a space if it is positive |
flag: -
String[] fullNames = new String[] {"Tom", "Jerry", "Donald"};
float[] salaries = new float[] {1000, 1500, 1200};
// s
System.out.printf("|%-10s | %-30s | %-15s |%n", "No", "Full Name", "Salary");
for(int i=0; i< fullNames.length; i++) {
System.out.printf("|%-10d | %-30s | %15f |%n", (i+1), fullNames[i], salaries[i]);
}
Output:
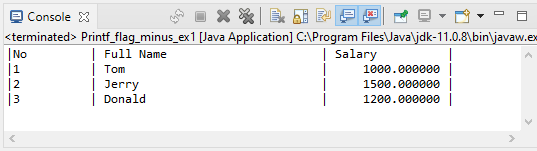
flag: +
// flag: +
System.out.printf("The world's economy increased by %+f percent in 2020. %n", -3.3);
System.out.printf("China's economy increased by %+f percent in 2020. %n", 2.3);
System.out.println();
// without flag: +
System.out.printf("The world's economy increased by %f percent in 2020. %n", -3.3);
System.out.printf("China's economy increased by %f percent in 2020. %n", 2.3);
Output:
The world's economy increased by -3.300000 percent in 2020.
China's economy increased by +2.300000 percent in 2020.
The world's economy increased by -3.300000 percent in 2020.
China's economy increased by 2.300000 percent in 2020.
flag: 0 (zero)
// flag: 0 & with: 20
System.out.printf("|%020f|%n", -3.1);
// without flag & with: 20
System.out.printf("|%20f|%n", -3.1);
// flag: - (left align) & with: 20
System.out.printf("|%-20f
Output:
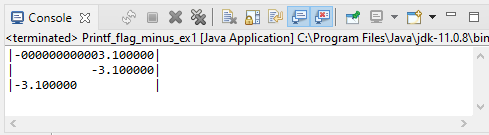
flag: , (comma)
// flag: ,
System.out.printf("%,f %n", 12345678.9);
// flag: ,
System.out.printf("%,f %n", -12345678.9);
Output:
12,345,678.900000
-12,345,678.900000
flag: (space)
// flag: (space)
System.out.printf("% f %n", 12345678.9);
System.out.printf("% f %n", -12345678.9);
System.out.println();
// flags: , (space + comma)
System.out.printf("% ,f %n", 12345678.9);
System.out.printf("% ,
Output:
12345678.900000
-12345678.900000
12,345,678.900000
-12,345,678.900000
6. precision
%[flags][width][.precision]conversion-character
System.out.printf("%f %n", 12345678.911);
System.out.printf("%f %n", -12345678.911);
System.out.println();
// flag: , (comma)
System.out.printf("%,f %n", 12345678.911);
// flag: , (comma)
System.out.printf("%,f %n", -12345678.911);
System.out.println();
// flags: , (space + comma)
System.out.printf("% ,f %n", 12345678.911);
// flags: , (space + comma)
System.out.printf("% ,f %n", -12345678.911);
System.out.println();
// flag: , (comma) & precision: .2
System.out.printf("%,.2f %n", 12345678.911);
// flag: , (comma) & precision: .3
System.out.printf("%,.3f %n", -12345678.911);
System.out.println();
// flags: , (space + comma) & precision: .2
System.out.printf("% ,.2f %n", 12345678.911);
// flags: , (space + comma) & precision: .3
System.out.printf("% ,.3f %n", -12345678.911);
Output:
12345678.911000
-12345678.911000
12,345,678.911000
-12,345,678.911000
12,345,678.911000
-12,345,678.911000
12,345,678.91
-12,345,678.911
12,345,678.91
-12,345,678.911
Java Basic
- Настройте java compiler для обработки вашего Annotation (Annotation Processing Tool)
- Программирование на Java для группы с помощью Eclipse и SVN
- Руководство Java WeakReference
- Руководство Java PhantomReference
- Сжатие и декомпрессия в Java
- Настройка Eclipse для использования JDK вместо JRE
- Методы String.format() и printf() в Java
- Синтаксис и новые функции в Java 8
- Регулярные выражения Java
- Руководство Java Multithreading Programming
- Библиотеки Java JDBC Driver для различных типов баз данных
- Руководство Java JDBC
- Получить значения столбцов, автоматически возрастающих при вставлении (Insert) записи, используя JDBC
- Руководство Java Stream
- Руководство Java Functional Interface
- Введение в Raspberry Pi
- Руководство Java Predicate
- Абстрактный класс и Interface в Java
- Модификатор доступа (Access modifiers) в Java
- Руководство Java Enum
- Руководство Java Annotation
- Сравнение и Сортировка в Java
- Руководство Java String, StringBuffer и StringBuilder
- Обработка исключений Java - Java Exception Handling
- Руководство Java Generics
- Манипулирование файлами и каталогами в Java
- Руководство Java BiPredicate
- Руководство Java Consumer
- Руководство Java BiConsumer
- Что мне нужно для начала работы с Java?
- История Java и разница между Oracle JDK и OpenJDK
- Установить Java в Windows
- Установите Java в Ubuntu
- Установите OpenJDK в Ubuntu
- Установить Eclipse
- Установите Eclipse в Ubuntu
- Быстрое изучение Java для начинающих
- История бит и байтов в информатике
- Типы данных в java
- Битовые операции
- Команда if else в Java
- команды switch в Java
- Циклы в Java
- Массивы (Array) в Java
- JDK Javadoc в формате CHM
- Наследование и полиморфизм в Java
- Руководство Java Function
- Руководство Java BiFunction
- Пример Java encoding и decoding с использованием Apache Base64
- Руководство Java Reflection
- Java Удаленный вызов методов - Java RMI
- Руководство Программирование Java Socket
- Какую платформу я должен выбрать для разработки приложений Java Desktop?
- Руководство Java Commons IO
- Руководство Java Commons Email
- Руководство Java Commons Logging
- Понимание Java System.identityHashCode, Object.hashCode и Object.equals
- Руководство Java SoftReference
- Руководство Java Supplier
- Аспектно-ориентированное программирование Java с помощью AspectJ (AOP)
Show More
- Руководства Java Servlet/JSP
- Руководства Java Collections Framework
- Java API для HTML, XML
- Руководства Java IO
- Руководства Java Date Time
- Руководства Spring Boot
- Руководства Maven
- Руководства Gradle
- Руководства Java Web Services
- Руководства Java SWT
- Руководства JavaFX
- Руководства Oracle Java ADF
- Руководства Struts2 Framework
- Руководства Spring Cloud