Циклы в JavaScript
1. Обзор циклов в ECMAScript
В ECMAScript команды (statement) выполняются последовательно сверху вниз. Однако, если вы хотите несколько раз выполнить блок команд, вы можете использовать цикл (loop).
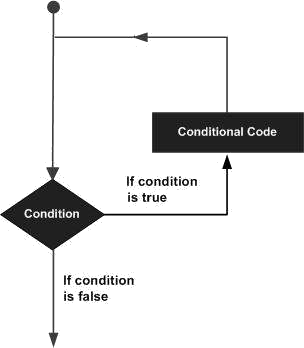
ECMAScript предоставляет вам 3 вида цикла:
- while loop
- for loop
- do .. while loop
Команды (statement) могут быть использованы внутри цикла:
- continue
- break
Команда | Описание |
break | Завершает оператор цикла. |
continue | Заставляет цикл пропустить остальные команды в блоке (block) и немедленно повторить проверку условий до повторения. |
2. Цикл while
Синтаксис цикла while:
while (condition) {
// Do something here
// ....
}
Пример:
while-loop-example.js
console.log("While loop example");
// Declare a variable, and assign value of 2.
let x = 2;
// Condition is x < 10
// If x < 10 is true then run block
while (x < 10) {
console.log("Value of x = ", x);
x = x + 3;
}
// This statment is out of while block.
console.log("Finish");
Output:
While loop example
Value of x = 2
Value of x = 5
Value of x = 8
Finish
3. Цикл for
Стандартный цикл for имеет следующий синтаксис:
for(InitialValues; condition; updateValues )
{
// Statements
}
- InitialValues: Инициализирует значения для связанных переменных в цикле.
- condition: Определяет условия для выполнения блока команд.
- updateValues: Обновляет новые значения для переменных.
for-loop-example.js
console.log("For loop example");
for( let i = 0; i < 10; i= i + 3) {
console.log("i= "+ i);
}
Output:
For loop example
i= 0
i= 3
i= 6
i= 9
for-loop-example2.js
console.log("For loop example");
for(let i = 0, j = 0; i + j < 10; i = i+1, j = j+2) {
console.log("i = " + i +", j = " + j);
}
Output:
For loop example
i = 0, j = 0
i = 1, j = 2
i = 2, j = 4
i = 3, j = 6
Использование цикла for может помочь вам просматривать на элементах массива.
for-loop-example3.js
console.log("For loop example");
// Array
let names =["Tom","Jerry", "Donald"];
for (let i = 0; i < names.length; i++ ) {
console.log("Name = ", names[i]);
}
console.log("End of example");
Output:
For loop example
Name = Tom
Name = Jerry
Name = Donald
End of example
4. Цикл for .. in
Цикл for..in помогает вам повторять property объекта.
for (prop in object) {
// Do something
}
Пример:
for-in-loop-example.js
// An object has 4 properties (name, age, gender,greeing)
var myObject = {
name : "John",
age: 25,
gender: "Male",
greeting : function() {
return "Hello";
}
};
for(myProp in myObject) {
console.log(myProp);
}
Output:
name
age
gender
greeting
5. Цикл for .. of
Цикл for..of помогает вам повторять на Collection (Коллекции), например Map, Set.
Пример:
for-of-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
for(let fruit of fruits) {
console.log(fruit);
}
Output:
Apple
Banana
Papaya
for-of-example2.js
// Create a Map object.
var myContacts = new Map();
myContacts.set("0100-1111", "Tom");
myContacts.set("0100-5555", "Jerry");
myContacts.set("0100-2222", "Donald");
for( let arr of myContacts) {
console.log(arr);
console.log(" - Phone: " + arr[0]);
console.log(" - Name: " + arr[1]);
}
Output:
[ '0100-1111', 'Tom' ]
- Phone: 0100-1111
- Name: Tom
[ '0100-5555', 'Jerry' ]
- Phone: 0100-5555
- Name: Jerry
[ '0100-2222', 'Donald' ]
- Phone: 0100-2222
- Name: Donald
6. Цикл do .. while
Цикл do-while используется для выполнения программы много раз. Особенностью do-while является то, что блок команд всегда выполняется минимум один раз. После каждого повтора (iteration), программа перепроверяет условие, если условие все еще является верным, блок команд снова выполнится.
do {
// Do something
}
while (expression);
Пример:
do-while-loop-example.js
let value = 3;
do {
console.log("Value = " + value);
value = value + 3;
} while (value < 10);
Output:
Value = 3
Value = 6
Value = 9
7. Использование команды break в цикле
break - это команда, которая может находиться блоке команд цикла. Этот команда завершает цикл безоговорочно.
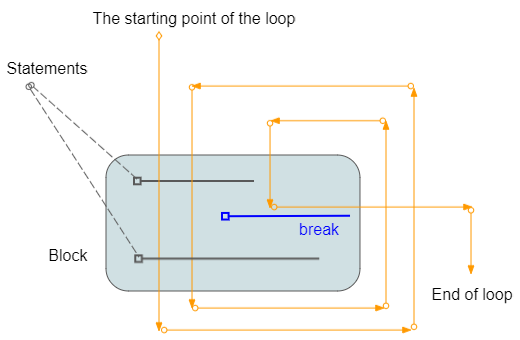
loop-break-example.js
console.log("Break example");
// Declare a variable and assign value of 2.
let x = 2;
while (x < 15) {
console.log("----------------------");
console.log("x = ", x);
// If x = 5 then exit the loop.
if (x == 5) {
break;
}
// Increase value of x by 1
x = x + 1;
console.log("x after + 1 = ", x);
}
console.log("End of example");
Output:
Break example
----------------------
x = 2
x after + 1 = 3
----------------------
x = 3
x after + 1 = 4
----------------------
x = 4
x after + 1 = 5
----------------------
x = 5
End of example
8. Использование команды continue в цикле
continue - это команда, которая может находиться в цикле. Когда встречается команда continue, программа проигнорирует командные строки в блоке ниже continue и начинает новый цикл.
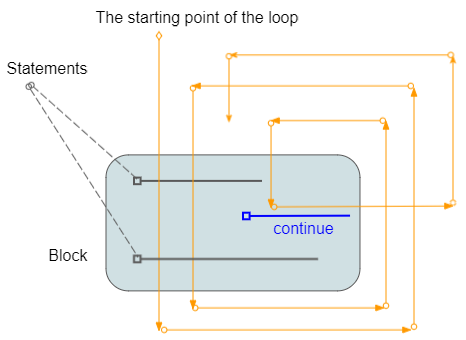
loop-continue-example.js
console.log("Continue example");
// Declare a variable and assign value of 2
x = 2
while (x < 7) {
console.log("----------------------")
console.log("x = ", x)
// % is used for calculating remainder
// If x is even, then ignore the command line below of continue
// and start new iteration.
if (x % 2 == 0) {
// Increase x by 1.
x = x + 1;
continue;
}
else {
// Increase x by 1.
x = x + 1
console.log("x after + 1 =", x)
}
}
console.log("End of example");
Output:
Continue example
----------------------
x = 2
----------------------
x = 3
x after + 1 = 4
----------------------
x = 4
----------------------
x = 5
x after + 1 = 6
----------------------
x = 6
End of example
9. Помеченный цикл (Labelled Loop)
ECMAScript позволяет вам прикрепить метку (Label) циклу, это похоже на то, что вы даете название циклу, это полезно когда вы используете много циклов сплетенных в одной программе.
- Вы можете использовать команду break labelX; чтобы break цикл с меткой labelX.
- Вы можете использовать команду continue labelX; чтобы continue цикл с меткой labelX.
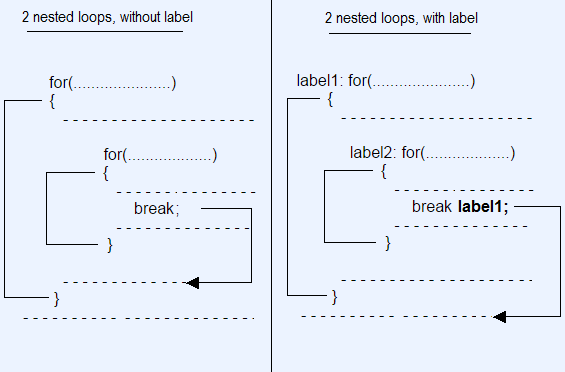
Синтаксис:
// for loop with Label.
label1: for( ... ) {
}
// while loop with Label.
label2: while ( ... ) {
}
// do-while loop with Label.
label3: do {
} while ( ... );
Пример использования сплетенных циклов, с меткой и отмеченной командой break.
labelled-loop-break-example.js
console.log("Labelled Loop Break example");
let i = 0;
label1: while (i < 5) {
console.log("----------------------\n");
console.log("i = " + i);
i++;
label2: for (let j = 0; j < 3; j++) {
console.log(" --> " + j);
if (j > 0) {
// Exit the loop with label1.
break label1;
}
}
}
console.log("Done!");
Output:
Labelled Loop Break example
----------------------
i = 0
--> 0
--> 1
Done!
Пример использования сплетенных циклов, с меткой и отмеченной командой continue.
labelled-loop-continue-example.js
let i = 0;
label1: while (i < 5) {
console.log("outer i= " + i);
i++;
label2: for (let j = 0; j < 3; j++) {
if (j > 0) {
continue label2;
}
if (i > 1) {
continue label1;
}
console.log("inner i= " + i + ", j= " + j);
}
}
Output:
outer i= 0
inner i= 1, j= 0
outer i= 1
outer i= 2
outer i= 3
outer i= 4
Pуководства ECMAScript, Javascript
- Введение в Javascript и ECMAScript
- Быстрый старт с Javascript
- Диалоговое окно Alert, Confirm, Prompt в Javascript
- Быстрый запуск с JavaScript
- Переменные (Variable) в JavaScript
- Битовые операции
- Массивы (Array) в JavaScript
- Циклы в JavaScript
- Руководство JavaScript Function
- Руководство JavaScript Number
- Руководство JavaScript Boolean
- Руководство JavaScript String
- Заявление if/else в JavaScript
- Заявление Switch в JavaScript
- Обработка ошибок в JavaScript
- Руководство JavaScript Date
- Руководство JavaScript Module
- Функция setTimeout и setInterval в JavaScript
- Руководство Javascript Form Validation
- Руководство JavaScript Web Cookie
- Ключевое слово void в JavaScript
- Классы и объекты в JavaScript
- Техника симулирования класса и наследственности в JavaScript
- Наследование и полиморфизм в JavaScript
- Понимание Duck Typing в JavaScript
- Руководство JavaScript Symbol
- Руководство JavaScript Set Collection
- Руководство JavaScript Map Collection
- Понимание JavaScript Iterable и Iterator
- Руководство Регулярное выражение JavaScript
- Руководство JavaScript Promise, Async Await
- Руководство Javascript Window
- Руководство Javascript Console
- Руководство Javascript Screen
- Руководство Javascript Navigator
- Руководство Javascript Geolocation API
- Руководство Javascript Location
- Руководство Javascript History API
- Руководство Javascript Statusbar
- Руководство Javascript Locationbar
- Руководство Javascript Scrollbars
- Руководство Javascript Menubar
- Руководство JavaScript JSON
- Обработка событий в Javascript
- Руководство Javascript MouseEvent
- Руководство Javascript WheelEvent
- Руководство Javascript KeyboardEvent
- Руководство Javascript FocusEvent
- Руководство Javascript InputEvent
- Руководство Javascript ChangeEvent
- Руководство Javascript DragEvent
- Руководство Javascript HashChangeEvent
- Руководство Javascript URL Encoding
- Руководство Javascript FileReader
- Руководство Javascript XMLHttpRequest
- Руководство Javascript Fetch API
- Разбор XML в Javascript с помощью DOMParser
- Введение в Javascript HTML5 Canvas API
- Выделение кода с помощью библиотеки Javascript SyntaxHighlighter
Show More