Руководство Android JSON Parser
1. Что таккое JSON?
JSON (JavaScript Object Notation) это формат обмена данных (data exchange format). Хранит данные по парам ключей и значения. По сравнению с XML, JSON проще для чтения.
Посмотрим простой пример JSON:
{
"name" : "Tran",
"address" : "Hai Duong, Vietnam",
"phones" : [0121111111, 012222222]
}
Пары ключей-значений (key-value) могут быть сплетены:
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
В Java имеется очень много библиотек с открытым испходным кодом, помогающие вам манипулировать с документами JSON, например:
- JSONP
- json.org
- Jackson
- Google GSON
- json-lib
- javax json
- json-simple
- json-smart
- flexjson
- fastjson
Android поддерживает готовые библиотеки для работы с JSON, вам не нужно объявлять никакую другую библиотеку. В данной статье я покажу вам как работать с JSON используя готовый JSON API в операционной системе Android.
3. Быстро создать Project для работы с JSON
Я быстро создаю проект с названием JSONTutorial для работы с JSON через примеры.
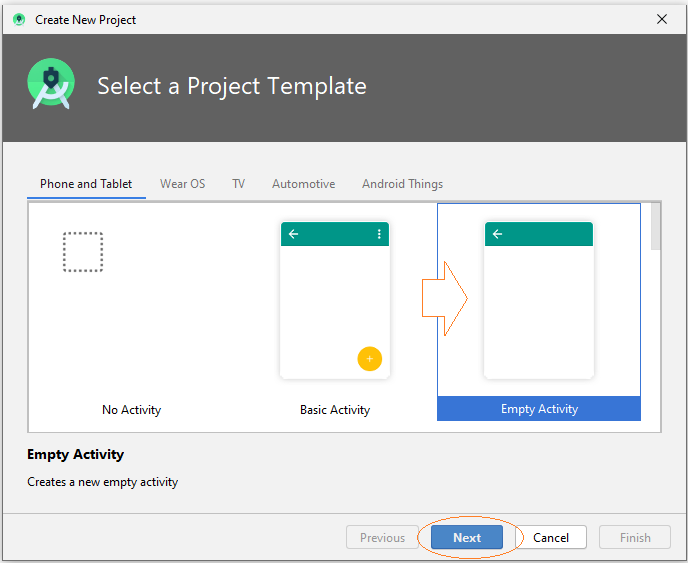
- Name: JSONTutorial
- Package name: org.o7planning.jsontutorial
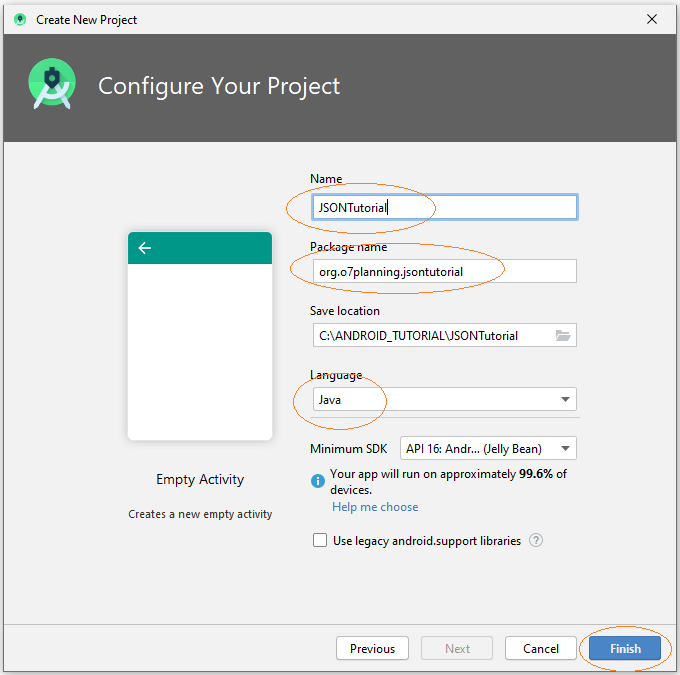
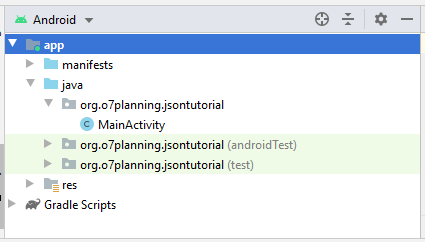
Создать папку raw:
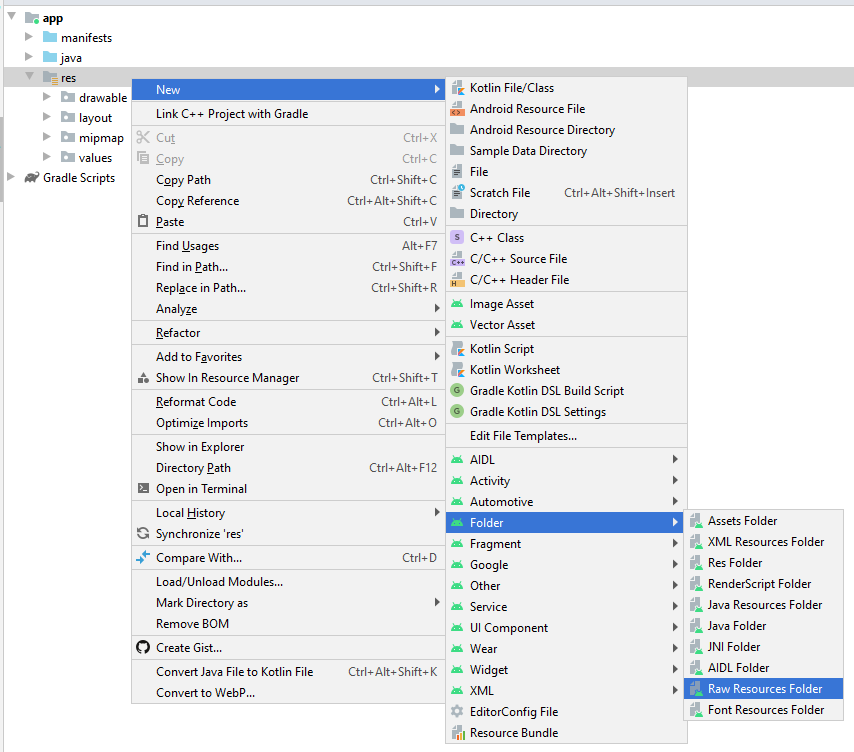
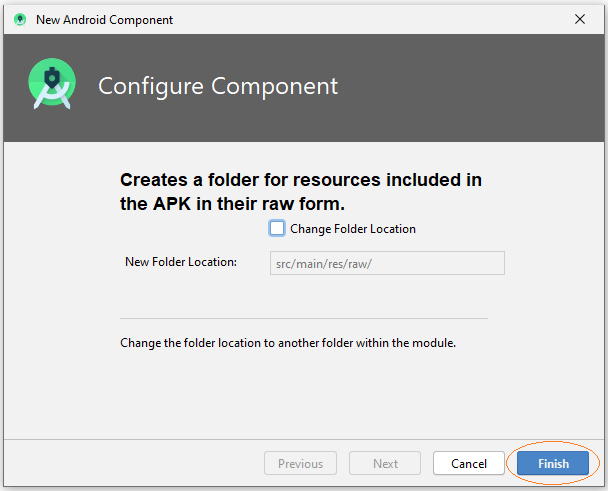
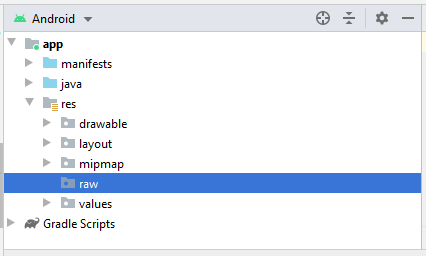
Я создаю еще несколько файлов json в папке 'raw', эти файлы будут участвовать в примерах данной статьи.
Ресурсы предоставляющие данные JSON могут быть от файла или URL,... В конце статьи вы можете посмотреть пример получения данных JSON из URL, анализированные и отображенные на приложении Android.
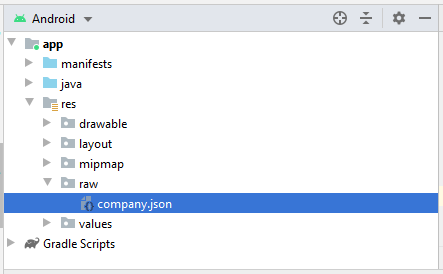
company.json
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
Дизайн activity_main.xml:
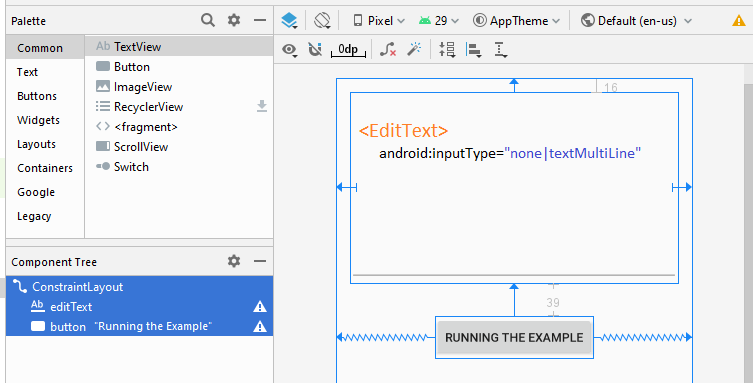
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="220dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="none|textMultiLine"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="39dp"
android:text="Running the Example"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
</androidx.constraintlayout.widget.ConstraintLayout>
4. Java Beans classes
Некоторые классы, участвующие в примерах:
Address.java
package org.o7planning.jsontutorial.beans;
public class Address {
private String street;
private String city;
public Address() {
}
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
@Override
public String toString() {
return street + ", " + city;
}
}
Company.java
package org.o7planning.jsontutorial.beans;
public class Company {
private int id;
private String name;
private String[] websites;
private Address address;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String[] getWebsites() {
return websites;
}
public void setWebsites(String[] websites) {
this.websites = websites;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("\n id:" + this.id);
sb.append("\n name:" + this.name);
if (this.websites != null) {
sb.append("\n website: ");
for (String website : this.websites) {
sb.append(website + ", ");
}
}
if (this.address != null) {
sb.append("\n address:" + this.address.toString());
}
return sb.toString();
}
}
5. Например прочитать данные JSON конвертированные в объект Java
В данном примере мы прочитаем файл данных JSON и конвертируем его в объект Java.
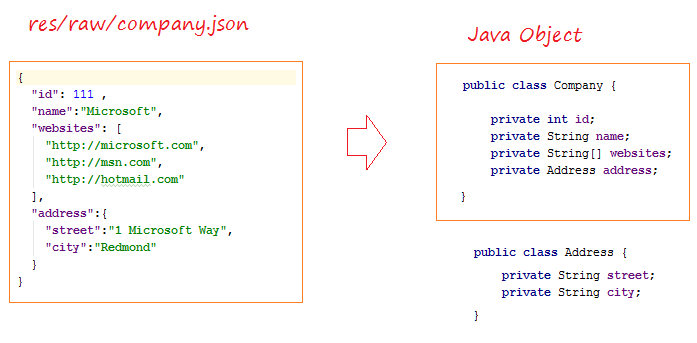
ReadJSONExample.java
package org.o7planning.jsontutorial.json;
import android.content.Context;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.o7planning.jsontutorial.R;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class ReadJSONExample {
// Read the company.json file and convert it to a java object.
public static Company readCompanyJSONFile(Context context) throws IOException,JSONException {
// Read content of company.json
String jsonText = readText(context, R.raw.company);
JSONObject jsonRoot = new JSONObject(jsonText);
int id= jsonRoot.getInt("id");
String name = jsonRoot.getString("name");
JSONArray jsonArray = jsonRoot.getJSONArray("websites");
String[] websites = new String[jsonArray.length()];
for(int i=0;i < jsonArray.length();i++) {
websites[i] = jsonArray.getString(i);
}
JSONObject jsonAddress = jsonRoot.getJSONObject("address");
String street = jsonAddress.getString("street");
String city = jsonAddress.getString("city");
Address address= new Address(street, city);
Company company = new Company();
company.setId(id);
company.setName(name);
company.setAddress(address);
company.setWebsites(websites);
return company;
}
private static String readText(Context context, int resId) throws IOException {
InputStream is = context.getResources().openRawResource(resId);
BufferedReader br= new BufferedReader(new InputStreamReader(is));
StringBuilder sb= new StringBuilder();
String s= null;
while(( s = br.readLine())!=null) {
sb.append(s);
sb.append("\n");
}
return sb.toString();
}
}
MainActivity.java
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.ReadJSONExample;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
Company company = ReadJSONExample.readCompanyJSONFile(this);
outputText.setText(company.toString());
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Запуск примера:
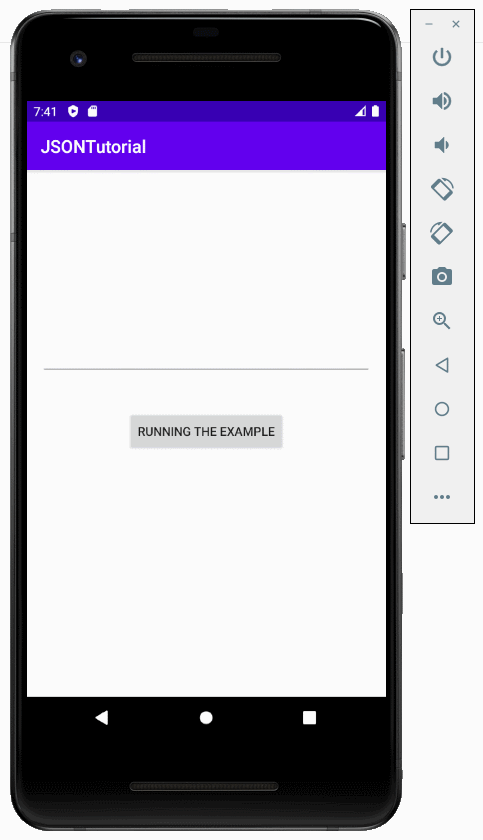
6. Пример ковертирования объекта Java в данные JSON
Следующий пример конвертирует объект Java в данные JSON.
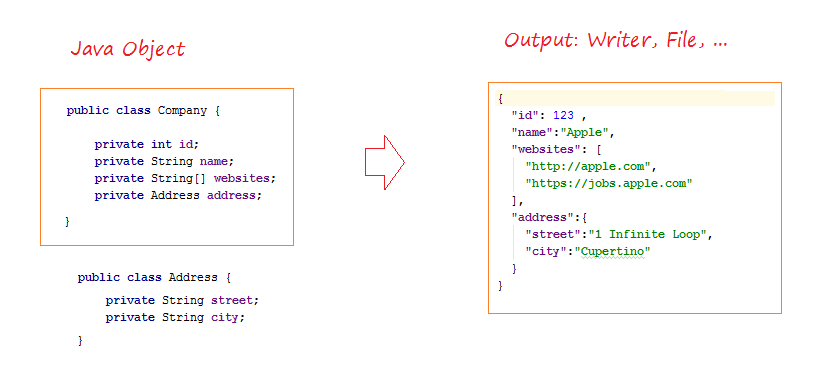
JsonWriterExample.java
package org.o7planning.jsontutorial.json;
import android.util.JsonWriter;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.IOException;
import java.io.Writer;
public class JsonWriterExample {
public static void writeJsonStream(Writer output, Company company ) throws IOException {
JsonWriter jsonWriter = new JsonWriter(output);
jsonWriter.beginObject();// begin root
jsonWriter.name("id").value(company.getId());
jsonWriter.name("name").value(company.getName());
String[] websites= company.getWebsites();
// "websites": [ ....]
jsonWriter.name("websites").beginArray(); // begin websites
for(String website: websites) {
jsonWriter.value(website);
}
jsonWriter.endArray();// end websites
// "address": { ... }
jsonWriter.name("address").beginObject(); // begin address
jsonWriter.name("street").value(company.getAddress().getStreet());
jsonWriter.name("city").value(company.getAddress().getCity());
jsonWriter.endObject();// end address
// end root
jsonWriter.endObject();
}
public static Company createCompany() {
Company company = new Company();
company.setId(123);
company.setName("Apple");
String[] websites = { "http://apple.com", "https://jobs.apple.com" };
company.setWebsites(websites);
Address address = new Address();
address.setCity("Cupertino");
address.setStreet("1 Infinite Loop");
company.setAddress(address);
return company;
}
}
MainActivity.java (2)
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.JsonWriterExample;
import java.io.StringWriter;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
StringWriter output = new StringWriter();
Company company = JsonWriterExample.createCompany();
JsonWriterExample.writeJsonStream(output, company);
String jsonText = output.toString();
outputText.setText(jsonText);
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Запуск приложения:
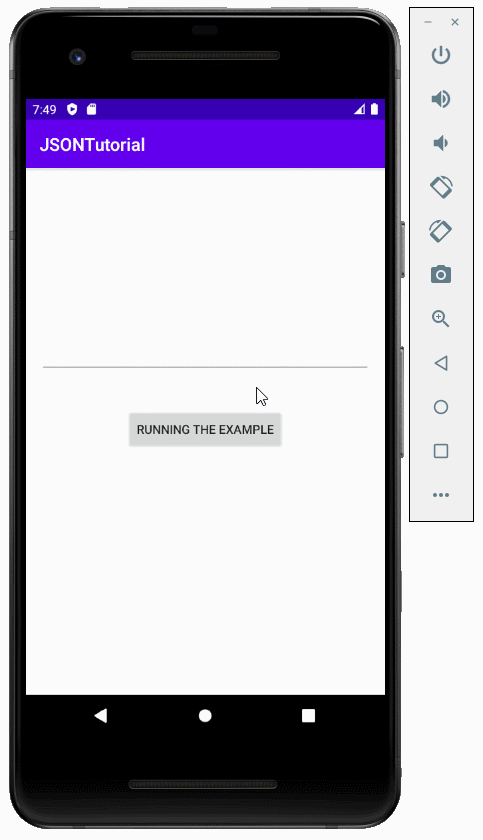
Pуководства Android
- Настроить Android Emulator в Android Studio
- Руководство Android ToggleButton
- Создать простой File Finder Dialog в Android
- Руководство Android TimePickerDialog
- Руководство Android DatePickerDialog
- Что мне нужно для начала работы с Android?
- Установите Android Studio в Windows
- Установите Intel® HAXM для Android Studio
- Руководство Android AsyncTask
- Руководство Android AsyncTaskLoader
- Руководство Android для начинающих - основные примеры
- Как узнать номер телефона Android Emulator и изменить его?
- Руководство Android TextInputLayout
- Руководство Android CardView
- Руководство Android ViewPager2
- Получить номер телефона в Android с помощью TelephonyManager
- Руководство Android Phone Call
- Руководство Android Wifi Scanning
- Руководство Android 2D Game для начинающих
- Руководство Android DialogFragment
- Руководство Android CharacterPickerDialog
- Руководство Android для начинающих - Hello Android
- Использование Android Device File Explorer
- Включить USB Debugging на устройстве Android
- Руководство Android UI Layouts
- Руководство Android SMS
- Руководство Android SQLite Database
- Руководство Google Maps Android API
- Руководство Текст в речь на Android
- Руководство Android Space
- Руководство Android Toast
- Создание пользовательских Android Toast
- Руководство Android SnackBar
- Руководство Android TextView
- Руководство Android TextClock
- Руководство Android EditText
- Руководство Android TextWatcher
- Форматирование номера кредитной карты с помощью Android TextWatcher
- Руководство Android Clipboard
- Создать простой File Chooser в Android
- Руководство Android AutoCompleteTextView и MultiAutoCompleteTextView
- Руководство Android ImageView
- Руководство Android ImageSwitcher
- Руководство Android ScrollView и HorizontalScrollView
- Руководство Android WebView
- Руководство Android SeekBar
- Руководство Android Dialog
- Руководство Android AlertDialog
- Руководство Android RatingBar
- Руководство Android ProgressBar
- Руководство Android Spinner
- Руководство Android Button
- Руководство Android Switch
- Руководство Android ImageButton
- Руководство Android FloatingActionButton
- Руководство Android CheckBox
- Руководство Android RadioGroup и RadioButton
- Руководство Android Chip и ChipGroup
- Использование Image assets и Icon assets Android Studio
- Настройка SD Card для Android Emulator
- Пример ChipGroup и Chip Entry
- Как добавить внешние библиотеки в Android Project в Android Studio?
- Как отключить разрешения, уже предоставленные приложению Android?
- Как удалить приложения из Android Emulator?
- Руководство Android LinearLayout
- Руководство Android TableLayout
- Руководство Android FrameLayout
- Руководство Android QuickContactBadge
- Руководство Android StackView
- Руководство Android Camera
- Руководство Android MediaPlayer
- Руководство Android VideoView
- Воспроизведение звуковых эффектов в Android с помощью SoundPool
- Руководство Android Networking
- Руководство Android JSON Parser
- Руководство Android SharedPreferences
- Руководство Android Internal Storage
- Руководство Android External Storage
- Руководство Android Intents
- Пример явного Android Intent, вызов другого Intent
- Пример неявного Android Intent, откройте URL, отправьте email
- Руководство Android Services
- Использовать оповещения в Android - Android Notification
- Руководство Android DatePicker
- Руководство Android TimePicker
- Руководство Android Chronometer
- Руководство Android OptionMenu
- Руководство Android ContextMenu
- Руководство Android PopupMenu
- Руководство Android Fragment
- Руководство Android ListView
- Android ListView с Checkbox с помощью ArrayAdapter
- Руководство Android GridView
Show More