Руководство Android SharedPreferences
1. Что такое SharedPreferences?
Для начала чтобы прояснить что такое SharedPreferences посмотрим на следующий случай:
Вы играете в игру на Android, перед тем как начать игру вы выбирете параметры игры например яркость экрана в игре, громкость звука, уровень сложности. После завершения вы можете выйти из игры и начать играть в следующий день. SharedPreferences позволяет вам сохранить выбранные до этого параметры, и позволяет вам переиграть без перенастройки.
Вы играете в игру на Android, перед тем как начать игру вы выбирете параметры игры например яркость экрана в игре, громкость звука, уровень сложности. После завершения вы можете выйти из игры и начать играть в следующий день. SharedPreferences позволяет вам сохранить выбранные до этого параметры, и позволяет вам переиграть без перенастройки.
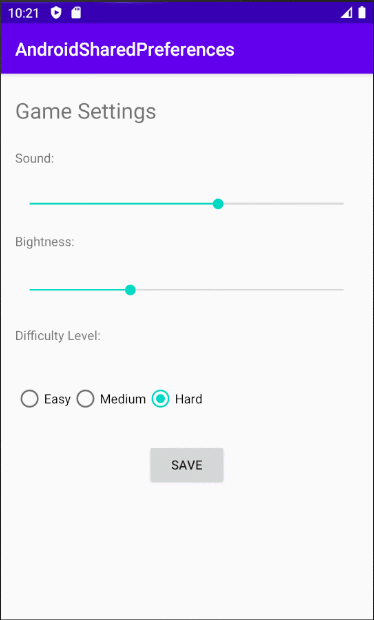
SharedPreferences сохраняет необработанные данные в виде пар ключей и значений (key-value) в папки приложения. Вы так же можете выбрать приватный режим сохранения (PRIVATE), который не позволит другим приложения иметь доступ к этим файлам и будет безопасным.
2. Пример с SharedPreferences
Иллюстрация интерфейса приложения:
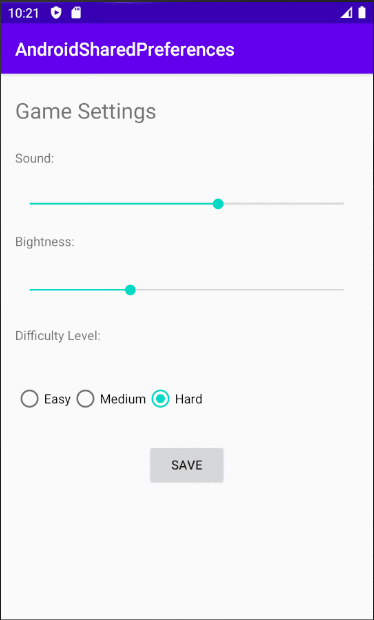
Создать новый project с названием AndroidSharedPreferences:
- File > New > New Project > Empty Activity
- Name: AndroidSharedPreferences
- Package name: org.o7planning.androidsharedpreferences
- Language: Java
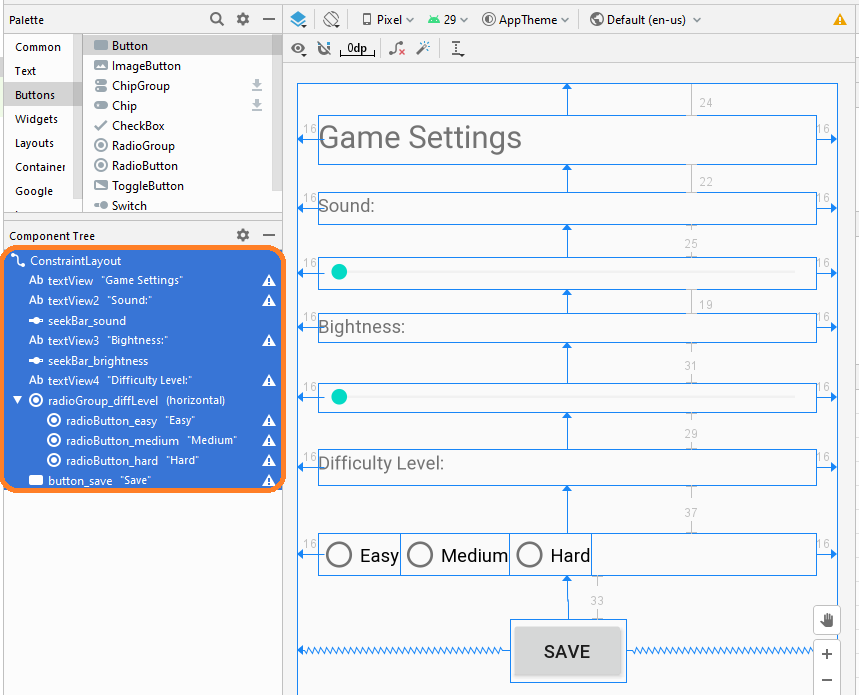
Если вас интересуют шаги дизайна интерфейса данного приложения, смотрите приложение в конце статьи.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="37dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Game Settings"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="22dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Sound:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<SeekBar
android:id="@+id/seekBar_sound"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="25dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<TextView
android:id="@+id/textView3"
android:layout_width="0dp"
android:layout_height="22dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Bightness:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar_sound" />
<SeekBar
android:id="@+id/seekBar_brightness"
android:layout_width="0dp"
android:layout_height="22dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="31dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView3" />
<TextView
android:id="@+id/textView4"
android:layout_width="0dp"
android:layout_height="27dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="29dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Difficulty Level:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar_brightness" />
<RadioGroup
android:id="@+id/radioGroup_diffLevel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="37dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView4">
<RadioButton
android:id="@+id/radioButton_easy"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Easy" />
<RadioButton
android:id="@+id/radioButton_medium"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Medium" />
<RadioButton
android:id="@+id/radioButton_hard"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hard" />
</RadioGroup>
<Button
android:id="@+id/button_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="33dp"
android:text="Save"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/radioGroup_diffLevel" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidsharedpreferences;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.SeekBar;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private SeekBar seekBarSound ;
private SeekBar seekBarBrightness;
private RadioGroup radioGroupDiffLevel;
private RadioButton radioButtonEasy;
private RadioButton radioButtonMedium;
private RadioButton radioButtonHard;
private Button buttonSave;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.seekBarBrightness= (SeekBar)this.findViewById(R.id.seekBar_brightness);
this.seekBarSound= (SeekBar)this.findViewById(R.id.seekBar_sound);
this.seekBarBrightness.setMax(100);
this.seekBarSound.setMax(100);
this.radioGroupDiffLevel= (RadioGroup) this.findViewById(R.id.radioGroup_diffLevel);
this.radioButtonEasy=(RadioButton) this.findViewById(R.id.radioButton_easy);
this.radioButtonMedium = (RadioButton)this.findViewById(R.id.radioButton_medium);
this.radioButtonHard=(RadioButton) this.findViewById(R.id.radioButton_hard);
this.buttonSave = (Button) this.findViewById(R.id.button_save);
this.buttonSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
MainActivity.this.doSave(view);
}
});
// Load saved game setting.
this.loadGameSetting();
}
private void loadGameSetting() {
SharedPreferences sharedPreferences= this.getSharedPreferences("gameSetting", Context.MODE_PRIVATE);
if(sharedPreferences!= null) {
int brightness = sharedPreferences.getInt("brightness", 90);
int sound = sharedPreferences.getInt("sound",95);
int checkedRadioButtonId = sharedPreferences.getInt("checkedRadioButtonId", R.id.radioButton_medium);
this.seekBarSound.setProgress(sound);
this.seekBarBrightness.setProgress(brightness);
this.radioGroupDiffLevel.check(checkedRadioButtonId);
} else {
this.radioGroupDiffLevel.check(R.id.radioButton_medium);
Toast.makeText(this,"Use the default game setting",Toast.LENGTH_LONG).show();
}
}
// Called when user click to Save button.
public void doSave(View view) {
// The created file can only be accessed by the calling application
// (or all applications sharing the same user ID).
SharedPreferences sharedPreferences= this.getSharedPreferences("gameSetting", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putInt("brightness", this.seekBarBrightness.getProgress());
editor.putInt("sound", this.seekBarSound.getProgress());
// Checked RadioButton ID.
int checkedRadioButtonId = radioGroupDiffLevel.getCheckedRadioButtonId();
editor.putInt("checkedRadioButtonId", checkedRadioButtonId);
// Save.
editor.apply();
Toast.makeText(this,"Game Setting saved!",Toast.LENGTH_LONG).show();
}
}
После сохранения, вы можете выйти из приложения, и открыть еще раз, сохраненные параметры установятся автоматически для игры.
Pуководства Android
- Настроить Android Emulator в Android Studio
- Руководство Android ToggleButton
- Создать простой File Finder Dialog в Android
- Руководство Android TimePickerDialog
- Руководство Android DatePickerDialog
- Что мне нужно для начала работы с Android?
- Установите Android Studio в Windows
- Установите Intel® HAXM для Android Studio
- Руководство Android AsyncTask
- Руководство Android AsyncTaskLoader
- Руководство Android для начинающих - основные примеры
- Как узнать номер телефона Android Emulator и изменить его?
- Руководство Android TextInputLayout
- Руководство Android CardView
- Руководство Android ViewPager2
- Получить номер телефона в Android с помощью TelephonyManager
- Руководство Android Phone Call
- Руководство Android Wifi Scanning
- Руководство Android 2D Game для начинающих
- Руководство Android DialogFragment
- Руководство Android CharacterPickerDialog
- Руководство Android для начинающих - Hello Android
- Использование Android Device File Explorer
- Включить USB Debugging на устройстве Android
- Руководство Android UI Layouts
- Руководство Android SMS
- Руководство Android SQLite Database
- Руководство Google Maps Android API
- Руководство Текст в речь на Android
- Руководство Android Space
- Руководство Android Toast
- Создание пользовательских Android Toast
- Руководство Android SnackBar
- Руководство Android TextView
- Руководство Android TextClock
- Руководство Android EditText
- Руководство Android TextWatcher
- Форматирование номера кредитной карты с помощью Android TextWatcher
- Руководство Android Clipboard
- Создать простой File Chooser в Android
- Руководство Android AutoCompleteTextView и MultiAutoCompleteTextView
- Руководство Android ImageView
- Руководство Android ImageSwitcher
- Руководство Android ScrollView и HorizontalScrollView
- Руководство Android WebView
- Руководство Android SeekBar
- Руководство Android Dialog
- Руководство Android AlertDialog
- Руководство Android RatingBar
- Руководство Android ProgressBar
- Руководство Android Spinner
- Руководство Android Button
- Руководство Android Switch
- Руководство Android ImageButton
- Руководство Android FloatingActionButton
- Руководство Android CheckBox
- Руководство Android RadioGroup и RadioButton
- Руководство Android Chip и ChipGroup
- Использование Image assets и Icon assets Android Studio
- Настройка SD Card для Android Emulator
- Пример ChipGroup и Chip Entry
- Как добавить внешние библиотеки в Android Project в Android Studio?
- Как отключить разрешения, уже предоставленные приложению Android?
- Как удалить приложения из Android Emulator?
- Руководство Android LinearLayout
- Руководство Android TableLayout
- Руководство Android FrameLayout
- Руководство Android QuickContactBadge
- Руководство Android StackView
- Руководство Android Camera
- Руководство Android MediaPlayer
- Руководство Android VideoView
- Воспроизведение звуковых эффектов в Android с помощью SoundPool
- Руководство Android Networking
- Руководство Android JSON Parser
- Руководство Android SharedPreferences
- Руководство Android Internal Storage
- Руководство Android External Storage
- Руководство Android Intents
- Пример явного Android Intent, вызов другого Intent
- Пример неявного Android Intent, откройте URL, отправьте email
- Руководство Android Services
- Использовать оповещения в Android - Android Notification
- Руководство Android DatePicker
- Руководство Android TimePicker
- Руководство Android Chronometer
- Руководство Android OptionMenu
- Руководство Android ContextMenu
- Руководство Android PopupMenu
- Руководство Android Fragment
- Руководство Android ListView
- Android ListView с Checkbox с помощью ArrayAdapter
- Руководство Android GridView
Show More